Table Of Contents
Spinner¶
New in version 1.4.0.
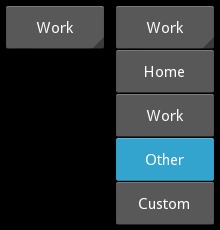
Spinner is a widget that provides a quick way to select one value from a set. In the default state, a spinner shows its currently selected value. Touching the spinner displays a dropdown menu with all the other available values from which the user can select a new one.
Example:
from kivy.base import runTouchApp
from kivy.uix.spinner import Spinner
spinner = Spinner(
# default value shown
text='Home',
# available values
values=('Home', 'Work', 'Other', 'Custom'),
# just for positioning in our example
size_hint=(None, None),
size=(100, 44),
pos_hint={'center_x': .5, 'center_y': .5})
def show_selected_value(spinner, text):
print('The spinner', spinner, 'has text', text)
spinner.bind(text=show_selected_value)
runTouchApp(spinner)
Kv Example:
FloatLayout:
Spinner:
size_hint: None, None
size: 100, 44
pos_hint: {'center': (.5, .5)}
text: 'Home'
values: 'Home', 'Work', 'Other', 'Custom'
on_text:
print("The spinner {} has text {}".format(self, self.text))
- class kivy.uix.spinner.Spinner(**kwargs)[source]¶
Bases:
kivy.uix.button.Button
Spinner class, see module documentation for more information.
- dropdown_cls¶
Class used to display the dropdown list when the Spinner is pressed.
dropdown_cls
is anObjectProperty
and defaults toDropDown
.Changed in version 1.8.0: If set to a string, the
Factory
will be used to resolve the class name.
- is_open¶
By default, the spinner is not open. Set to True to open it.
is_open
is aBooleanProperty
and defaults to False.New in version 1.4.0.
- option_cls¶
Class used to display the options within the dropdown list displayed under the Spinner. The text property of the class will be used to represent the value.
The option class requires:
a text property, used to display the value.
an on_release event, used to trigger the option when pressed/touched.
a
size_hint_y
of None.the
height
to be set.
option_cls
is anObjectProperty
and defaults toSpinnerOption
.Changed in version 1.8.0: If you set a string, the
Factory
will be used to resolve the class.
- sync_height¶
Each element in a dropdown list uses a default/user-supplied height. Set to True to propagate the Spinner’s height value to each dropdown list element.
New in version 1.10.0.
sync_height
is aBooleanProperty
and defaults to False.
- text_autoupdate¶
Indicates if the spinner’s
text
should be automatically updated with the first value of thevalues
property. Setting it to True will cause the spinner to update itstext
property every time attr:values are changed.New in version 1.10.0.
text_autoupdate
is aBooleanProperty
and defaults to False.
- values¶
Values that can be selected by the user. It must be a list of strings.
values
is aListProperty
and defaults to [].
- class kivy.uix.spinner.SpinnerOption(**kwargs)[source]¶
Bases:
kivy.uix.button.Button
Special button used in the
Spinner
dropdown list. By default, this is just aButton
with a size_hint_y of None and a height of48dp
.