Table Of Contents
- Scatter
- Usage
- Control Interactions
- Automatic Bring to Front
- Scale Limitation
- Behavior
Scatter
Scatter.apply_transform()
Scatter.auto_bring_to_front
Scatter.bbox
Scatter.center
Scatter.center_x
Scatter.center_y
Scatter.collide_point()
Scatter.do_collide_after_children
Scatter.do_rotation
Scatter.do_scale
Scatter.do_translation
Scatter.do_translation_x
Scatter.do_translation_y
Scatter.on_bring_to_front()
Scatter.on_motion()
Scatter.on_touch_down()
Scatter.on_touch_move()
Scatter.on_touch_up()
Scatter.on_transform_with_touch()
Scatter.pos
Scatter.right
Scatter.rotation
Scatter.scale
Scatter.scale_max
Scatter.scale_min
Scatter.to_local()
Scatter.to_parent()
Scatter.top
Scatter.transform
Scatter.transform_inv
Scatter.translation_touches
Scatter.x
Scatter.y
ScatterPlane
Scatter¶
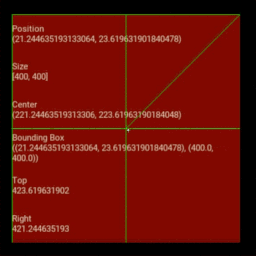
Scatter
is used to build interactive widgets that can be translated,
rotated and scaled with two or more fingers on a multitouch system.
Scatter has its own matrix transformation: the modelview matrix is changed before the children are drawn and the previous matrix is restored when the drawing is finished. That makes it possible to perform rotation, scaling and translation over the entire children tree without changing any widget properties. That specific behavior makes the scatter unique, but there are some advantages / constraints that you should consider:
The children are positioned relative to the scatter similarly to a
RelativeLayout
. So when dragging the scatter, the position of the children don’t change, only the position of the scatter does.The scatter size has no impact on the size of its children.
If you want to resize the scatter, use scale, not size (read #2). Scale transforms both the scatter and its children, but does not change size.
The scatter is not a layout. You must manage the size of the children yourself.
For touch events, the scatter converts from the parent matrix to the scatter
matrix automatically in on_touch_down/move/up events. If you are doing things
manually, you will need to use to_parent()
and
to_local()
.
Usage¶
By default, the Scatter does not have a graphical representation: it is a
container only. The idea is to combine the Scatter with another widget, for
example an Image
:
scatter = Scatter()
image = Image(source='sun.jpg')
scatter.add_widget(image)
Control Interactions¶
By default, all interactions are enabled. You can selectively disable them using the do_rotation, do_translation and do_scale properties.
Disable rotation:
scatter = Scatter(do_rotation=False)
Allow only translation:
scatter = Scatter(do_rotation=False, do_scale=False)
Allow only translation on x axis:
scatter = Scatter(do_rotation=False, do_scale=False,
do_translation_y=False)
Automatic Bring to Front¶
If the Scatter.auto_bring_to_front
property is True, the scatter
widget will be removed and re-added to the parent when it is touched
(brought to front, above all other widgets in the parent). This is useful
when you are manipulating several scatter widgets and don’t want the active
one to be partially hidden.
Scale Limitation¶
We are using a 32-bit matrix in double representation. That means we have a limit for scaling. You cannot do infinite scaling down/up with our implementation. Generally, you don’t hit the minimum scale (because you don’t see it on the screen), but the maximum scale is 9.99506983235e+19 (2^66).
You can also limit the minimum and maximum scale allowed:
scatter = Scatter(scale_min=.5, scale_max=3.)
Behavior¶
Changed in version 1.1.0: If no control interactions are enabled, then the touch handler will never return True.
- class kivy.uix.scatter.Scatter(**kwargs)[source]¶
Bases:
kivy.uix.widget.Widget
Scatter class. See module documentation for more information.
- Events:
- on_transform_with_touch:
Fired when the scatter has been transformed by user touch or multitouch, such as panning or zooming.
- on_bring_to_front:
Fired when the scatter is brought to the front.
Changed in version 1.9.0: Event on_bring_to_front added.
Changed in version 1.8.0: Event on_transform_with_touch added.
- apply_transform(trans, post_multiply=False, anchor=(0, 0))[source]¶
Transforms the scatter by applying the “trans” transformation matrix (on top of its current transformation state). The resultant matrix can be found in the
transform
property.- Parameters:
- trans:
Matrix
. Transformation matrix to be applied to the scatter widget.
- anchor: tuple, defaults to (0, 0).
The point to use as the origin of the transformation (uses local widget space).
- post_multiply: bool, defaults to False.
If True, the transform matrix is post multiplied (as if applied before the current transform).
- trans:
Usage example:
from kivy.graphics.transformation import Matrix mat = Matrix().scale(3, 3, 3) scatter_instance.apply_transform(mat)
- auto_bring_to_front¶
If True, the widget will be automatically pushed on the top of parent widget list for drawing.
auto_bring_to_front
is aBooleanProperty
and defaults to True.
- bbox¶
Bounding box of the widget in parent space:
((x, y), (w, h)) # x, y = lower left corner
bbox
is anAliasProperty
.
- center¶
Center position of the widget.
center
is aReferenceListProperty
of (center_x
,center_y
) properties.
- center_x¶
X center position of the widget.
center_x
is anAliasProperty
of (x
+width
/ 2.).
- center_y¶
Y center position of the widget.
center_y
is anAliasProperty
of (y
+height
/ 2.).
- collide_point(x, y)[source]¶
Check if a point (x, y) is inside the widget’s axis aligned bounding box.
- Parameters:
- x: numeric
x position of the point (in parent coordinates)
- y: numeric
y position of the point (in parent coordinates)
- Returns:
A bool. True if the point is inside the bounding box, False otherwise.
>>> Widget(pos=(10, 10), size=(50, 50)).collide_point(40, 40) True
- do_collide_after_children¶
If True, the collision detection for limiting the touch inside the scatter will be done after dispaching the touch to the children. You can put children outside the bounding box of the scatter and still be able to touch them.
do_collide_after_children
is aBooleanProperty
and defaults to False.New in version 1.3.0.
- do_rotation¶
Allow rotation.
do_rotation
is aBooleanProperty
and defaults to True.
- do_scale¶
Allow scaling.
do_scale
is aBooleanProperty
and defaults to True.
- do_translation¶
Allow translation on the X or Y axis.
do_translation
is anAliasProperty
of (do_translation_x
+do_translation_y
)
- do_translation_x¶
Allow translation on the X axis.
do_translation_x
is aBooleanProperty
and defaults to True.
- do_translation_y¶
Allow translation on Y axis.
do_translation_y
is aBooleanProperty
and defaults to True.
- on_bring_to_front(touch)[source]¶
Called when a touch event causes the scatter to be brought to the front of the parent (only if
auto_bring_to_front
is True)- Parameters:
- touch:
The touch object which brought the scatter to front.
New in version 1.9.0.
- on_motion(etype, me)[source]¶
Called when a motion event is received.
- Parameters:
- etype: str
Event type, one of “begin”, “update” or “end”
- me:
MotionEvent
Received motion event
- Returns:
bool True to stop event dispatching
New in version 2.1.0.
Warning
This is an experimental method and it remains so while this warning is present.
- on_touch_down(touch)[source]¶
Receive a touch down event.
- Parameters:
- touch:
MotionEvent
class Touch received. The touch is in parent coordinates. See
relativelayout
for a discussion on coordinate systems.
- touch:
- Returns:
bool If True, the dispatching of the touch event will stop. If False, the event will continue to be dispatched to the rest of the widget tree.
- on_touch_move(touch)[source]¶
Receive a touch move event. The touch is in parent coordinates.
See
on_touch_down()
for more information.
- on_touch_up(touch)[source]¶
Receive a touch up event. The touch is in parent coordinates.
See
on_touch_down()
for more information.
- on_transform_with_touch(touch)[source]¶
Called when a touch event has transformed the scatter widget. By default this does nothing, but can be overridden by derived classes that need to react to transformations caused by user input.
- Parameters:
- touch:
The touch object which triggered the transformation.
New in version 1.8.0.
- pos¶
Position of the widget.
pos
is aReferenceListProperty
of (x
,y
) properties.
- right¶
Right position of the widget.
right
is anAliasProperty
of (x
+width
).
- rotation¶
Rotation value of the scatter in degrees moving in a counterclockwise direction.
rotation
is anAliasProperty
and defaults to 0.0.
- scale¶
Scale value of the scatter.
scale
is anAliasProperty
and defaults to 1.0.
- scale_max¶
Maximum scaling factor allowed.
scale_max
is aNumericProperty
and defaults to 1e20.
- scale_min¶
Minimum scaling factor allowed.
scale_min
is aNumericProperty
and defaults to 0.01.
- to_local(x, y, **k)[source]¶
Transform parent coordinates to local (current widget) coordinates.
See
relativelayout
for details on the coordinate systems.- Parameters:
- relative: bool, defaults to False
Change to True if you want to translate coordinates to relative widget coordinates.
- to_parent(x, y, **k)[source]¶
Transform local (current widget) coordinates to parent coordinates.
See
relativelayout
for details on the coordinate systems.- Parameters:
- relative: bool, defaults to False
Change to True if you want to translate relative positions from a widget to its parent coordinates.
- top¶
Top position of the widget.
top
is anAliasProperty
of (y
+height
).
- transform¶
Transformation matrix.
transform
is anObjectProperty
and defaults to the identity matrix.Note
This matrix reflects the current state of the transformation matrix but setting it directly will erase previously applied transformations. To apply a transformation considering context, please use the
apply_transform
method.
- transform_inv¶
Inverse of the transformation matrix.
transform_inv
is anObjectProperty
and defaults to the identity matrix.
- translation_touches¶
Determine whether translation was triggered by a single or multiple touches. This only has effect when
do_translation
= True.translation_touches
is aNumericProperty
and defaults to 1.New in version 1.7.0.
- x¶
X position of the widget.
x
is aNumericProperty
and defaults to 0.
- y¶
Y position of the widget.
y
is aNumericProperty
and defaults to 0.
- class kivy.uix.scatter.ScatterPlane(**kwargs)[source]¶
Bases:
kivy.uix.scatter.Scatter
This is essentially an unbounded Scatter widget. It’s a convenience class to make it easier to handle infinite planes.
- collide_point(x, y)[source]¶
Check if a point (x, y) is inside the widget’s axis aligned bounding box.
- Parameters:
- x: numeric
x position of the point (in parent coordinates)
- y: numeric
y position of the point (in parent coordinates)
- Returns:
A bool. True if the point is inside the bounding box, False otherwise.
>>> Widget(pos=(10, 10), size=(50, 50)).collide_point(40, 40) True