Table Of Contents
Box Layout¶
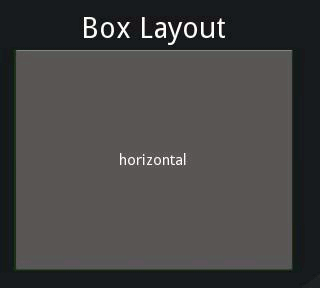
BoxLayout
arranges children in a vertical or horizontal box.
To position widgets above/below each other, use a vertical BoxLayout:
layout = BoxLayout(orientation='vertical')
btn1 = Button(text='Hello')
btn2 = Button(text='World')
layout.add_widget(btn1)
layout.add_widget(btn2)
To position widgets next to each other, use a horizontal BoxLayout. In this example, we use 10 pixel spacing between children; the first button covers 70% of the horizontal space, the second covers 30%:
layout = BoxLayout(spacing=10)
btn1 = Button(text='Hello', size_hint=(.7, 1))
btn2 = Button(text='World', size_hint=(.3, 1))
layout.add_widget(btn1)
layout.add_widget(btn2)
Position hints are partially working, depending on the orientation:
If the orientation is vertical: x, right and center_x will be used.
If the orientation is horizontal: y, top and center_y will be used.
Kv Example:
BoxLayout:
orientation: 'vertical'
Label:
text: 'this on top'
Label:
text: 'this right aligned'
size_hint_x: None
size: self.texture_size
pos_hint: {'right': 1}
Label:
text: 'this on bottom'
You can check the examples/widgets/boxlayout_poshint.py for a live example.
Note
The size_hint uses the available space after subtracting all the fixed-size widgets. For example, if you have a layout that is 800px wide, and add three buttons like this:
btn1 = Button(text='Hello', size=(200, 100), size_hint=(None, None))
btn2 = Button(text='Kivy', size_hint=(.5, 1))
btn3 = Button(text='World', size_hint=(.5, 1))
The first button will be 200px wide as specified, the second and third will be 300px each, e.g. (800-200) * 0.5
Changed in version 1.4.1: Added support for pos_hint.
- class kivy.uix.boxlayout.BoxLayout(**kwargs)[source]¶
Bases:
kivy.uix.layout.Layout
Box layout class. See module documentation for more information.
- add_widget(widget, *args, **kwargs)[source]¶
Add a new widget as a child of this widget.
- Parameters:
- widget:
Widget
Widget to add to our list of children.
- index: int, defaults to 0
Index to insert the widget in the list. Notice that the default of 0 means the widget is inserted at the beginning of the list and will thus be drawn on top of other sibling widgets. For a full discussion of the index and widget hierarchy, please see the Widgets Programming Guide.
New in version 1.0.5.
- canvas: str, defaults to None
Canvas to add widget’s canvas to. Can be ‘before’, ‘after’ or None for the default canvas.
New in version 1.9.0.
- widget:
>>> from kivy.uix.button import Button >>> from kivy.uix.slider import Slider >>> root = Widget() >>> root.add_widget(Button()) >>> slider = Slider() >>> root.add_widget(slider)
- do_layout(*largs)[source]¶
This function is called when a layout is called by a trigger. If you are writing a new Layout subclass, don’t call this function directly but use
_trigger_layout()
instead.The function is by default called before the next frame, therefore the layout isn’t updated immediately. Anything depending on the positions of e.g. children should be scheduled for the next frame.
New in version 1.0.8.
- minimum_height¶
Automatically computed minimum height needed to contain all children.
New in version 1.10.0.
minimum_height
is aNumericProperty
and defaults to 0. It is read only.
- minimum_size¶
Automatically computed minimum size needed to contain all children.
New in version 1.10.0.
minimum_size
is aReferenceListProperty
of (minimum_width
,minimum_height
) properties. It is read only.
- minimum_width¶
Automatically computed minimum width needed to contain all children.
New in version 1.10.0.
minimum_width
is aNumericProperty
and defaults to 0. It is read only.
- orientation¶
Orientation of the layout.
orientation
is anOptionProperty
and defaults to ‘horizontal’. Can be ‘vertical’ or ‘horizontal’.
- padding¶
Padding between layout box and children: [padding_left, padding_top, padding_right, padding_bottom].
padding also accepts a two argument form [padding_horizontal, padding_vertical] and a one argument form [padding].
Changed in version 1.7.0: Replaced NumericProperty with VariableListProperty.
padding
is aVariableListProperty
and defaults to [0, 0, 0, 0].
- remove_widget(widget, *args, **kwargs)[source]¶
Remove a widget from the children of this widget.
- Parameters:
- widget:
Widget
Widget to remove from our children list.
- widget:
>>> from kivy.uix.button import Button >>> root = Widget() >>> button = Button() >>> root.add_widget(button) >>> root.remove_widget(button)
- spacing¶
Spacing between children, in pixels.
spacing
is aNumericProperty
and defaults to 0.