Table Of Contents
- Carousel
Carousel
Carousel.add_widget()
Carousel.anim_cancel_duration
Carousel.anim_move_duration
Carousel.anim_type
Carousel.clear_widgets()
Carousel.current_slide
Carousel.direction
Carousel.ignore_perpendicular_swipes
Carousel.index
Carousel.load_next()
Carousel.load_previous()
Carousel.load_slide()
Carousel.loop
Carousel.min_move
Carousel.next_slide
Carousel.on_touch_down()
Carousel.on_touch_move()
Carousel.on_touch_up()
Carousel.previous_slide
Carousel.remove_widget()
Carousel.scroll_distance
Carousel.scroll_timeout
Carousel.slides
Carousel¶
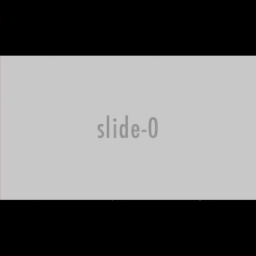
New in version 1.4.0.
The Carousel
widget provides the classic mobile-friendly carousel view
where you can swipe between slides.
You can add any content to the carousel and have it move horizontally or
vertically. The carousel can display pages in a sequence or a loop.
Example:
from kivy.app import App
from kivy.uix.carousel import Carousel
from kivy.uix.image import AsyncImage
class CarouselApp(App):
def build(self):
carousel = Carousel(direction='right')
for i in range(10):
src = "http://placehold.it/480x270.png&text=slide-%d&.png" % i
image = AsyncImage(source=src, fit_mode="contain")
carousel.add_widget(image)
return carousel
CarouselApp().run()
Kv Example:
Carousel:
direction: 'right'
AsyncImage:
source: 'http://placehold.it/480x270.png&text=slide-1.png'
AsyncImage:
source: 'http://placehold.it/480x270.png&text=slide-2.png'
AsyncImage:
source: 'http://placehold.it/480x270.png&text=slide-3.png'
AsyncImage:
source: 'http://placehold.it/480x270.png&text=slide-4.png'
Changed in version 1.5.0: The carousel now supports active children, like the
ScrollView
. It will detect a swipe gesture
according to the Carousel.scroll_timeout
and
Carousel.scroll_distance
properties.
In addition, the slide container is no longer exposed by the API.
The impacted properties are
Carousel.slides
, Carousel.current_slide
,
Carousel.previous_slide
and Carousel.next_slide
.
- class kivy.uix.carousel.Carousel(**kwargs)[source]¶
Bases:
kivy.uix.stencilview.StencilView
Carousel class. See module documentation for more information.
- add_widget(widget, index=0, *args, **kwargs)[source]¶
Add a new widget as a child of this widget.
- Parameters:
- widget:
Widget
Widget to add to our list of children.
- index: int, defaults to 0
Index to insert the widget in the list. Notice that the default of 0 means the widget is inserted at the beginning of the list and will thus be drawn on top of other sibling widgets. For a full discussion of the index and widget hierarchy, please see the Widgets Programming Guide.
New in version 1.0.5.
- canvas: str, defaults to None
Canvas to add widget’s canvas to. Can be ‘before’, ‘after’ or None for the default canvas.
New in version 1.9.0.
- widget:
>>> from kivy.uix.button import Button >>> from kivy.uix.slider import Slider >>> root = Widget() >>> root.add_widget(Button()) >>> slider = Slider() >>> root.add_widget(slider)
- anim_cancel_duration¶
Defines the duration of the animation when a swipe movement is not accepted. This is generally when the user does not make a large enough swipe. See
min_move
.anim_cancel_duration
is aNumericProperty
and defaults to 0.3.
- anim_move_duration¶
Defines the duration of the Carousel animation between pages.
anim_move_duration
is aNumericProperty
and defaults to 0.5.
- anim_type¶
Type of animation to use while animating to the next/previous slide. This should be the name of an
AnimationTransition
function.anim_type
is aStringProperty
and defaults to ‘out_quad’.New in version 1.8.0.
- clear_widgets(children=None, *args, **kwargs)[source]¶
Remove all (or the specified)
children
of this widget. If the ‘children’ argument is specified, it should be a list (or filtered list) of children of the current widget.Changed in version 1.8.0: The children argument can be used to specify the children you want to remove.
Changed in version 2.1.0: Specifying an empty
children
list leaves the widgets unchanged. Previously it was treated likeNone
and all children were removed.
- current_slide¶
The currently shown slide.
current_slide
is anAliasProperty
.Changed in version 1.5.0: The property no longer exposes the slides container. It returns the widget you have added.
- direction¶
Specifies the direction in which the slides are ordered. This corresponds to the direction from which the user swipes to go from one slide to the next. It can be right, left, top, or bottom. For example, with the default value of right, the second slide is to the right of the first and the user would swipe from the right towards the left to get to the second slide.
direction
is anOptionProperty
and defaults to ‘right’.
- ignore_perpendicular_swipes¶
Ignore swipes on axis perpendicular to direction.
ignore_perpendicular_swipes
is aBooleanProperty
and defaults to False.New in version 1.10.0.
- index¶
Get/Set the current slide based on the index.
index
is anAliasProperty
and defaults to 0 (the first item).
- load_slide(slide)[source]¶
Animate to the slide that is passed as the argument.
Changed in version 1.8.0.
- loop¶
Allow the Carousel to loop infinitely. If True, when the user tries to swipe beyond last page, it will return to the first. If False, it will remain on the last page.
loop
is aBooleanProperty
and defaults to False.
- min_move¶
Defines the minimum distance to be covered before the touch is considered a swipe gesture and the Carousel content changed. This is a expressed as a fraction of the Carousel’s width. If the movement doesn’t reach this minimum value, the movement is cancelled and the content is restored to its original position.
min_move
is aNumericProperty
and defaults to 0.2.
- next_slide¶
The next slide in the Carousel. It is None if the current slide is the last slide in the Carousel. This ordering reflects the order in which the slides are added: their presentation varies according to the
direction
property.next_slide
is anAliasProperty
.Changed in version 1.5.0: The property no longer exposes the slides container. It returns the widget you have added.
- on_touch_down(touch)[source]¶
Receive a touch down event.
- Parameters:
- touch:
MotionEvent
class Touch received. The touch is in parent coordinates. See
relativelayout
for a discussion on coordinate systems.
- touch:
- Returns:
bool If True, the dispatching of the touch event will stop. If False, the event will continue to be dispatched to the rest of the widget tree.
- on_touch_move(touch)[source]¶
Receive a touch move event. The touch is in parent coordinates.
See
on_touch_down()
for more information.
- on_touch_up(touch)[source]¶
Receive a touch up event. The touch is in parent coordinates.
See
on_touch_down()
for more information.
- previous_slide¶
The previous slide in the Carousel. It is None if the current slide is the first slide in the Carousel. This ordering reflects the order in which the slides are added: their presentation varies according to the
direction
property.previous_slide
is anAliasProperty
.Changed in version 1.5.0: This property no longer exposes the slides container. It returns the widget you have added.
- remove_widget(widget, *args, **kwargs)[source]¶
Remove a widget from the children of this widget.
- Parameters:
- widget:
Widget
Widget to remove from our children list.
- widget:
>>> from kivy.uix.button import Button >>> root = Widget() >>> button = Button() >>> root.add_widget(button) >>> root.remove_widget(button)
- scroll_distance¶
Distance to move before scrolling the
Carousel
in pixels. As soon as the distance has been traveled, theCarousel
will start to scroll, and no touch event will go to children. It is advisable that you base this value on the dpi of your target device’s screen.scroll_distance
is aNumericProperty
and defaults to 20dp.New in version 1.5.0.
- scroll_timeout¶
Timeout allowed to trigger the
scroll_distance
, in milliseconds. If the user has not movedscroll_distance
within the timeout, no scrolling will occur and the touch event will go to the children.scroll_timeout
is aNumericProperty
and defaults to 200 (milliseconds)New in version 1.5.0.
- slides¶
List of slides inside the Carousel. The slides are the widgets added to the Carousel using the
add_widget
method.slides
is aListProperty
and is read-only.