Table Of Contents
Float Layout¶
FloatLayout
honors the pos_hint
and the size_hint
properties of its children.
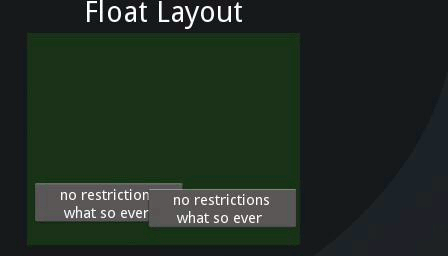
For example, a FloatLayout with a size of (300, 300) is created:
layout = FloatLayout(size=(300, 300))
By default, all widgets have their size_hint=(1, 1), so this button will adopt the same size as the layout:
button = Button(text='Hello world')
layout.add_widget(button)
To create a button 50% of the width and 25% of the height of the layout and positioned at (20, 20), you can do:
button = Button(
text='Hello world',
size_hint=(.5, .25),
pos=(20, 20))
If you want to create a button that will always be the size of layout minus 20% on each side:
button = Button(text='Hello world', size_hint=(.6, .6),
pos_hint={'x':.2, 'y':.2})
Note
This layout can be used for an application. Most of the time, you will use the size of Window.
Warning
If you are not using pos_hint, you must handle the positioning of the children: if the float layout is moving, you must handle moving the children too.
- class kivy.uix.floatlayout.FloatLayout(**kwargs)[source]¶
Bases:
kivy.uix.layout.Layout
Float layout class. See module documentation for more information.
- add_widget(widget, *args, **kwargs)[source]¶
Add a new widget as a child of this widget.
- Parameters:
- widget:
Widget
Widget to add to our list of children.
- index: int, defaults to 0
Index to insert the widget in the list. Notice that the default of 0 means the widget is inserted at the beginning of the list and will thus be drawn on top of other sibling widgets. For a full discussion of the index and widget hierarchy, please see the Widgets Programming Guide.
New in version 1.0.5.
- canvas: str, defaults to None
Canvas to add widget’s canvas to. Can be ‘before’, ‘after’ or None for the default canvas.
New in version 1.9.0.
- widget:
>>> from kivy.uix.button import Button >>> from kivy.uix.slider import Slider >>> root = Widget() >>> root.add_widget(Button()) >>> slider = Slider() >>> root.add_widget(slider)
- do_layout(*largs, **kwargs)[source]¶
This function is called when a layout is called by a trigger. If you are writing a new Layout subclass, don’t call this function directly but use
_trigger_layout()
instead.The function is by default called before the next frame, therefore the layout isn’t updated immediately. Anything depending on the positions of e.g. children should be scheduled for the next frame.
New in version 1.0.8.
- remove_widget(widget, *args, **kwargs)[source]¶
Remove a widget from the children of this widget.
- Parameters:
- widget:
Widget
Widget to remove from our children list.
- widget:
>>> from kivy.uix.button import Button >>> root = Widget() >>> button = Button() >>> root.add_widget(button) >>> root.remove_widget(button)