Table Of Contents
BoxShadow¶
New in version 2.2.0.
BoxShadow is a graphical instruction used to add a shadow effect to an element.
Its behavior is similar to the concept of a CSS3 box-shadow.
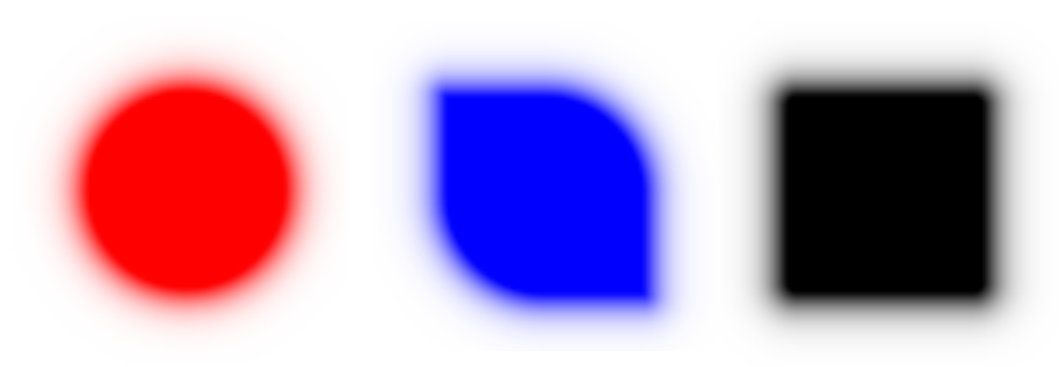
The BoxShadow declaration must occur inside a Canvas
statement. It works
similarly to other graphical instructions such as Rectangle
,
RoundedRectangle
, etc.
Note
Although the BoxShadow
graphical instruction has a visually similar behavior to box-shadow (CSS), the hierarchy
of the drawing layer of BoxShadow
in relation to the target element must be defined following the same layer
hierarchy rules as when declaring other canvas instructions.
For more details, refer to the inset
mode.
Example:¶
<MyWidget>: Button: pos_hint: {"center_x": 0.5, "center_y": 0.5} size_hint: None, None size: 200, 150 background_down: self.background_normal canvas.before: Color: rgba: 0, 0, 1, 0.85 BoxShadow: pos: self.pos size: self.size offset: 0, -10 spread_radius: -20, -20 border_radius: 10, 10, 10, 10 blur_radius: 80 if self.state == "normal" else 50
- class kivy.graphics.boxshadow.BoxShadow(*args, **kwargs)¶
Bases:
kivy.graphics.instructions.InstructionGroup
New in version 2.2.0.
Changed in version 2.3.0: Fixed
Canvas
management usingadd()
,insert()
andremove()
. Previously, using them to manage theCanvas
had no effect.The base class also changed from
Fbo
toInstructionGroup
.- Parameters:
- inset: bool, defaults to
False
. Defines whether the shadow is drawn from the inside out or from the outline to the inside of the
BoxShadow
instruction.- size: list | tuple, defaults to
(100.0, 100.0)
. Define the raw size of the shadow, that is, you should not take into account changes in the value of
blur_radius
andspread_radius
properties when setting this parameter.- pos: list | tuple, defaults to
(0.0, 0.0)
. Define the raw position of the shadow, that is, you should not take into account changes in the value of the
offset
property when setting this parameter.- offset: list | tuple, defaults to
(0.0, 0.0)
. Specifies shadow offsets in (horizontal, vertical) format. Positive values for the offset indicate that the shadow should move to the right and/or top. The negative ones indicate that the shadow should move to the left and/or down.
- blur_radius: float, defaults to
15.0
. Define the shadow blur radius. Controls shadow expansion and softness.
- spread_radius: list | tuple, defaults to
(0.0, 0.0)
. Define the shrink/expansion of the shadow.
- border_radius: list | tuple, defaults to
(0.0, 0.0, 0.0, 0.0)
. Specifies the radii used for the rounded corners clockwise: top-left, top-right, bottom-right, bottom-left.
- inset: bool, defaults to
- blur_radius¶
Define the shadow blur radius. Controls shadow expansion and softness.
Defaults to
15.0
.In the images below, the start and end positions of the shadow blur effect length are indicated. The transition between color and transparency is seamless, and although the shadow appears to end before before the dotted rectangle, its end is made to be as smooth as possible.
inset
OFF:
inset
ON:
Note
In some cases (if this is not your intention), placing an element above the shadow (before the blur radius ends) will result in a unwanted cropping/overlay behavior rather than continuity, breaking the shadow’s soft ending, as shown in the image below.
- border_radius¶
Specifies the radii used for the rounded corners clockwise: top-left, top-right, bottom-right, bottom-left.
Defaults to
(0.0, 0.0, 0.0, 0.0)
.inset
OFF:
inset
ON:
- inset¶
Defines whether the shadow is drawn from the inside out or from the outline to the inside of the
BoxShadow
instruction.Defaults to
False
.Note
Although the inset mode determines the drawing behavior of the shadow, the position of the
BoxShadow
instruction in thecanvas
hierarchy depends on the other graphic instructions present in theCanvas
instruction tree.In other words, if the target is in the
canvas
layer and you want to use the defaultinset = False
mode to create an elevation effect, you must declare theBoxShadow
instruction incanvas.before
layer.<MyWidget@Widget>: size_hint: None, None size: 100, 100 pos: 100, 100 canvas.before: # BoxShadow statements Color: rgba: 0, 0, 0, 0.65 BoxShadow: pos: self.pos size: self.size offset: 0, -10 blur_radius: 25 spread_radius: -10, -10 border_radius: 10, 10, 10, 10 canvas: # target element statements Color: rgba: 1, 1, 1, 1 Rectangle: pos: self.pos size: self.size
Or, if the target is in the
canvas
layer and you want to use theinset = True
mode to create an insertion effect, you must declare theBoxShadow
instruction in thecanvas
layer, immediately after the targetcanvas
declaration, or declare it incanvas.after
.<MyWidget@Widget>: size_hint: None, None size: 100, 100 pos: 100, 100 canvas: # target element statements Color: rgba: 1, 1, 1, 1 Rectangle: pos: self.pos size: self.size # BoxShadow statements Color: rgba: 0, 0, 0, 0.65 BoxShadow: inset: True pos: self.pos size: self.size offset: 0, -10 blur_radius: 25 spread_radius: -10, -10 border_radius: 10, 10, 10, 10
In summary:
Elevation effect -
inset = False
: theBoxShadow
instruction needs to be drawn before the target element.Insertion effect -
inset = True
: theBoxShadow
instruction needs to be drawn after the target element.
In general,
BoxShadow
is more flexible than box-shadow (CSS) because theinset = False
andinset = True
modes do not limit the drawing of the shadow below and above the target element, respectively. Actually, you can define any hierarchy you want in theCanvas
declaration tree, to create more complex effects that go beyond common shadow effects.Modes:
False
(default) - The shadow is drawn inside out theBoxShadow
instruction, creating a raised effect.True
- The shadow is drawn from the outline to the inside of theBoxShadow
instruction, creating a inset effect.
- offset¶
Specifies shadow offsets in [horizontal, vertical] format. Positive values for the offset indicate that the shadow should move to the right and/or top. The negative ones indicate that the shadow should move to the left and/or down.
Defaults to
(0.0, 0.0)
.For this property to work as expected, it is indicated that the value of
pos
coincides with the position of the target element of the shadow, as in the example below:inset
OFF:
inset
ON:
- pos¶
Define the raw position of the shadow, that is, you should not take into account changes in the value of the
offset
property when setting this property.inset
ON:Returns the raw position (the same as specified).
Defaults to
(0.0, 0.0)
.Note
It is recommended that this property matches the raw position of the shadow target element. To manipulate horizontal and vertical offset, use
offset
instead.
- size¶
Define the raw size of the shadow, that is, you should not take into account changes in the value of
blur_radius
andspread_radius
properties.inset
OFF:Returns the adjusted size of the shadow according to the
blur_radius
andspread_radius
properties.
inset
ON:Returns the raw size (the same as specified).
Defaults to
(100.0, 100.0)
.Note
It is recommended that this property matches the raw size of the shadow target element. To control the shrink/expansion of the shadow’s raw
size
, usespread_radius
instead.
- spread_radius¶
Define the shrink/expansion of the shadow in [horizontal, vertical] format.
Defaults to
(0.0, 0.0)
.This property is especially useful for cases where you want to achieve a softer shadow around the element, by setting negative values for
spread_radius
and a larger value forblur_radius
as in the example.inset
OFF:In the image below, the target element has a raw size of
200 x 150px
. Positive changes to thespread_radius
values will cause the rawsize
of the shadow to increase, while negative values will cause the shadow to shrink.
inset
ON:Positive values will cause the shadow to grow into the bounding box, while negative values will cause the shadow to shrink.