Table Of Contents
Basic Picture Viewer¶
This simple image browser demonstrates the scatter widget. You should see three framed photographs on a background. You can click and drag the photos around, or multi-touch to drop a red dot to scale and rotate the photos.
The photos are loaded from the local images directory, while the background picture is from the data shipped with kivy in kivy/data/images/background.jpg. The file pictures.kv describes the interface and the file shadow32.png is the border to make the images look like framed photographs. Finally, the file android.txt is used to package the application for use with the Kivy Launcher Android application.
For Android devices, you can copy/paste this directory into /sdcard/kivy/pictures on your Android device.
The images in the image directory are from the Internet Archive, https://archive.org/details/PublicDomainImages, and are in the public domain.
File demo/pictures/main.py¶
'''
Basic Picture Viewer
====================
This simple image browser demonstrates the scatter widget. You should
see three framed photographs on a background. You can click and drag
the photos around, or multi-touch to drop a red dot to scale and rotate the
photos.
The photos are loaded from the local images directory, while the background
picture is from the data shipped with kivy in kivy/data/images/background.jpg.
The file pictures.kv describes the interface and the file shadow32.png is
the border to make the images look like framed photographs. Finally,
the file android.txt is used to package the application for use with the
Kivy Launcher Android application.
For Android devices, you can copy/paste this directory into
/sdcard/kivy/pictures on your Android device.
The images in the image directory are from the Internet Archive,
`https://archive.org/details/PublicDomainImages`, and are in the public
domain.
'''
import kivy
kivy.require('1.0.6')
from glob import glob
from random import randint
from os.path import join, dirname
from kivy.app import App
from kivy.logger import Logger
from kivy.uix.scatter import Scatter
from kivy.properties import StringProperty
class Picture(Scatter):
'''Picture is the class that will show the image with a white border and a
shadow. They are nothing here because almost everything is inside the
picture.kv. Check the rule named <Picture> inside the file, and you'll see
how the Picture() is really constructed and used.
The source property will be the filename to show.
'''
source = StringProperty(None)
class PicturesApp(App):
def build(self):
# the root is created in pictures.kv
root = self.root
# get any files into images directory
curdir = dirname(__file__)
for filename in glob(join(curdir, 'images', '*')):
try:
# load the image
picture = Picture(source=filename, rotation=randint(-30, 30))
# add to the main field
root.add_widget(picture)
except Exception as e:
Logger.exception('Pictures: Unable to load <%s>' % filename)
def on_pause(self):
return True
if __name__ == '__main__':
PicturesApp().run()
File demo/pictures/pictures.kv¶
1#:kivy 1.0
2#:import kivy kivy
3#:import win kivy.core.window
4
5FloatLayout:
6 canvas:
7 Color:
8 rgb: 1, 1, 1
9 Rectangle:
10 source: 'data/images/background.jpg'
11 size: self.size
12
13 BoxLayout:
14 padding: 10
15 spacing: 10
16 size_hint: 1, None
17 pos_hint: {'top': 1}
18 height: 44
19 Image:
20 size_hint: None, None
21 size: 24, 24
22 source: 'data/logo/kivy-icon-24.png'
23 Label:
24 height: 24
25 text_size: self.width, None
26 color: (1, 1, 1, .8)
27 text: 'Kivy %s - Pictures' % kivy.__version__
28
29
30
31<Picture>:
32 # each time a picture is created, the image can delay the loading
33 # as soon as the image is loaded, ensure that the center is changed
34 # to the center of the screen.
35 on_size: self.center = win.Window.center
36 size: image.size
37 size_hint: None, None
38
39 Image:
40 id: image
41 source: root.source
42
43 # create initial image to be 400 pixels width
44 size: 400, 400 / self.image_ratio
45
46 # add shadow background
47 canvas.before:
48 Color:
49 rgba: 1,1,1,1
50 BorderImage:
51 source: 'shadow32.png'
52 border: (36,36,36,36)
53 size:(self.width+72, self.height+72)
54 pos: (-36,-36)
Image demo/pictures/shadow32.png¶
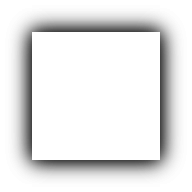
File demo/pictures/android.txt¶
1title=Pictures
2author=Kivy team
3orientation=landscape