Table Of Contents
- FileChooser
- Simple widgets
- Widget composition
- Usage example
FileChooser
FileChooserController
FileChooserController.cancel()
FileChooserController.dirselect
FileChooserController.entry_released()
FileChooserController.entry_touched()
FileChooserController.file_encodings
FileChooserController.file_system
FileChooserController.files
FileChooserController.filter_dirs
FileChooserController.filters
FileChooserController.font_name
FileChooserController.get_nice_size()
FileChooserController.layout
FileChooserController.multiselect
FileChooserController.on_touch_down()
FileChooserController.on_touch_up()
FileChooserController.path
FileChooserController.progress_cls
FileChooserController.rootpath
FileChooserController.selection
FileChooserController.show_hidden
FileChooserController.sort_func
FileChooserIconLayout
FileChooserIconView
FileChooserListLayout
FileChooserListView
FileChooserProgressBase
FileSystemAbstract
FileSystemLocal
FileChooser¶
The FileChooser module provides various classes for describing, displaying and browsing file systems.
Simple widgets¶
There are two ready-to-use widgets that provide views of the file system. Each of these present the files and folders in a different style.
The FileChooserListView
displays file entries as text items in a
vertical list, where folders can be collapsed and expanded.
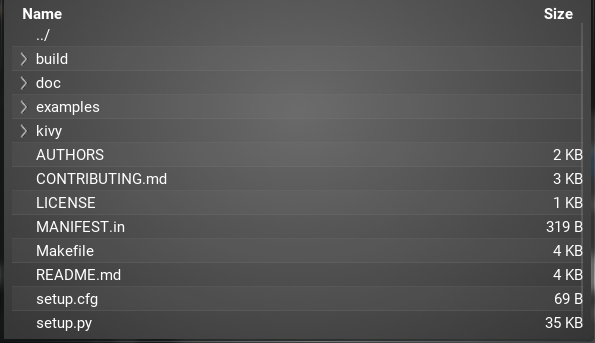
The FileChooserIconView
presents icons and text from left to right,
wrapping them as required.
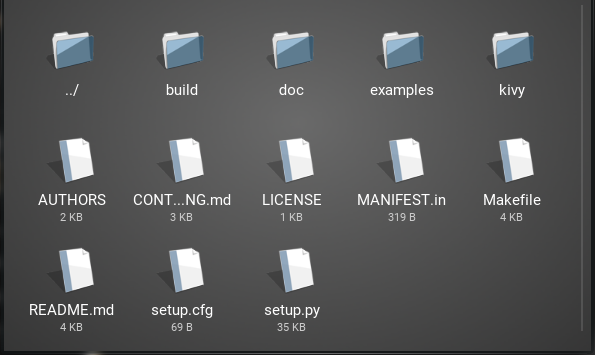
They both provide for scrolling, selection and basic user interaction.
Please refer to the FileChooserController
for details on supported
events and properties.
Widget composition¶
FileChooser classes adopt a MVC design. They are exposed so that you to extend and customize your file chooser according to your needs.
The FileChooser classes can be categorized as follows:
Models are represented by concrete implementations of the
FileSystemAbstract
class, such as theFileSystemLocal
.Views are represented by the
FileChooserListLayout
andFileChooserIconLayout
classes. These are used by theFileChooserListView
andFileChooserIconView
widgets respectively.Controllers are represented by concrete implementations of the
FileChooserController
, namely theFileChooser
,FileChooserIconView
andFileChooserListView
classes.
This means you can define your own views or provide FileSystemAbstract
implementations for alternative file systems for use with these widgets.
The FileChooser
can be used as a controller for handling multiple,
synchronized views of the same path. By combining these elements, you can add
your own views and file systems and have them easily interact with the existing
components.
Usage example¶
main.py
from kivy.app import App
from kivy.uix.floatlayout import FloatLayout
from kivy.factory import Factory
from kivy.properties import ObjectProperty
from kivy.uix.popup import Popup
import os
class LoadDialog(FloatLayout):
load = ObjectProperty(None)
cancel = ObjectProperty(None)
class SaveDialog(FloatLayout):
save = ObjectProperty(None)
text_input = ObjectProperty(None)
cancel = ObjectProperty(None)
class Root(FloatLayout):
loadfile = ObjectProperty(None)
savefile = ObjectProperty(None)
text_input = ObjectProperty(None)
def dismiss_popup(self):
self._popup.dismiss()
def show_load(self):
content = LoadDialog(load=self.load, cancel=self.dismiss_popup)
self._popup = Popup(title="Load file", content=content,
size_hint=(0.9, 0.9))
self._popup.open()
def show_save(self):
content = SaveDialog(save=self.save, cancel=self.dismiss_popup)
self._popup = Popup(title="Save file", content=content,
size_hint=(0.9, 0.9))
self._popup.open()
def load(self, path, filename):
with open(os.path.join(path, filename[0])) as stream:
self.text_input.text = stream.read()
self.dismiss_popup()
def save(self, path, filename):
with open(os.path.join(path, filename), 'w') as stream:
stream.write(self.text_input.text)
self.dismiss_popup()
class Editor(App):
pass
Factory.register('Root', cls=Root)
Factory.register('LoadDialog', cls=LoadDialog)
Factory.register('SaveDialog', cls=SaveDialog)
if __name__ == '__main__':
Editor().run()
editor.kv
#:kivy 1.1.0
Root:
text_input: text_input
BoxLayout:
orientation: 'vertical'
BoxLayout:
size_hint_y: None
height: 30
Button:
text: 'Load'
on_release: root.show_load()
Button:
text: 'Save'
on_release: root.show_save()
BoxLayout:
TextInput:
id: text_input
text: ''
RstDocument:
text: text_input.text
show_errors: True
<LoadDialog>:
BoxLayout:
size: root.size
pos: root.pos
orientation: "vertical"
FileChooserListView:
id: filechooser
BoxLayout:
size_hint_y: None
height: 30
Button:
text: "Cancel"
on_release: root.cancel()
Button:
text: "Load"
on_release: root.load(filechooser.path, filechooser.selection)
<SaveDialog>:
text_input: text_input
BoxLayout:
size: root.size
pos: root.pos
orientation: "vertical"
FileChooserListView:
id: filechooser
on_selection: text_input.text = self.selection and self.selection[0] or ''
TextInput:
id: text_input
size_hint_y: None
height: 30
multiline: False
BoxLayout:
size_hint_y: None
height: 30
Button:
text: "Cancel"
on_release: root.cancel()
Button:
text: "Save"
on_release: root.save(filechooser.path, text_input.text)
New in version 1.0.5.
Changed in version 1.2.0: In the chooser template, the controller is no longer a direct reference but a weak-reference. If you are upgrading, you should change the notation root.controller.xxx to root.controller().xxx.
- class kivy.uix.filechooser.FileChooser(**kwargs)[source]¶
Bases:
kivy.uix.filechooser.FileChooserController
Implementation of a
FileChooserController
which supports switching between multiple, synced layout views.The FileChooser can be used as follows:
BoxLayout: orientation: 'vertical' BoxLayout: size_hint_y: None height: sp(52) Button: text: 'Icon View' on_press: fc.view_mode = 'icon' Button: text: 'List View' on_press: fc.view_mode = 'list' FileChooser: id: fc FileChooserIconLayout FileChooserListLayout
New in version 1.9.0.
- add_widget(widget, *args, **kwargs)[source]¶
Add a new widget as a child of this widget.
- Parameters:
- widget:
Widget
Widget to add to our list of children.
- index: int, defaults to 0
Index to insert the widget in the list. Notice that the default of 0 means the widget is inserted at the beginning of the list and will thus be drawn on top of other sibling widgets. For a full discussion of the index and widget hierarchy, please see the Widgets Programming Guide.
New in version 1.0.5.
- canvas: str, defaults to None
Canvas to add widget’s canvas to. Can be ‘before’, ‘after’ or None for the default canvas.
New in version 1.9.0.
- widget:
>>> from kivy.uix.button import Button >>> from kivy.uix.slider import Slider >>> root = Widget() >>> root.add_widget(Button()) >>> slider = Slider() >>> root.add_widget(slider)
- manager¶
Reference to the
ScreenManager
instance.manager is an
ObjectProperty
.
- view_list¶
List of views added to this FileChooser.
view_list is an
AliasProperty
of typelist
.
- view_mode¶
Current layout view mode.
view_mode is an
AliasProperty
of typestr
.
- class kivy.uix.filechooser.FileChooserController(**kwargs)[source]¶
Bases:
kivy.uix.relativelayout.RelativeLayout
Base for implementing a FileChooser. Don’t use this class directly, but prefer using an implementation such as the
FileChooser
,FileChooserListView
orFileChooserIconView
.- Events:
- on_entry_added: entry, parent
Fired when a root-level entry is added to the file list. If you return True from this event, the entry is not added to FileChooser.
- on_entries_cleared
Fired when the the entries list is cleared, usually when the root is refreshed.
- on_subentry_to_entry: entry, parent
Fired when a sub-entry is added to an existing entry or when entries are removed from an entry e.g. when a node is closed.
- on_submit: selection, touch
Fired when a file has been selected with a double-tap.
- cancel(*largs)[source]¶
Cancel any background action started by filechooser, such as loading a new directory.
New in version 1.2.0.
- dirselect¶
Determines whether directories are valid selections or not.
dirselect is a
BooleanProperty
and defaults to False.New in version 1.1.0.
- entry_released(entry, touch)[source]¶
(internal) This method must be called by the template when an entry is touched by the user.
New in version 1.1.0.
- entry_touched(entry, touch)[source]¶
(internal) This method must be called by the template when an entry is touched by the user.
- file_encodings¶
Possible encodings for decoding a filename to unicode. In the case that the user has a non-ascii filename, undecodable without knowing its initial encoding, we have no other choice than to guess it.
Please note that if you encounter an issue because of a missing encoding here, we’ll be glad to add it to this list.
file_encodings is a
ListProperty
and defaults to [‘utf-8’, ‘latin1’, ‘cp1252’].New in version 1.3.0.
Deprecated since version 1.8.0: This property is no longer used as the filechooser no longer decodes the file names.
- file_system¶
The file system object used to access the file system. This should be a subclass of
FileSystemAbstract
.file_system is an
ObjectProperty
and defaults toFileSystemLocal()
New in version 1.8.0.
- files¶
The list of files in the directory specified by path after applying the filters.
files is a read-only
ListProperty
.
- filter_dirs¶
Indicates whether filters should also apply to directories. filter_dirs is a
BooleanProperty
and defaults to False.
- filters¶
filters specifies the filters to be applied to the files in the directory. filters is a
ListProperty
and defaults to []. This is equivalent to ‘*’ i.e. nothing is filtered.The filters are not reset when the path changes. You need to do that yourself if desired.
There are two kinds of filters: patterns and callbacks.
Patterns
e.g. [’*.png’]. You can use the following patterns:
Pattern
Meaning
*
matches everything
?
matches any single character
[seq]
matches any character in seq
[!seq]
matches any character not in seq
Callbacks
You can specify a function that will be called for each file. The callback will be passed the folder and file name as the first and second parameters respectively. It should return True to indicate a match and False otherwise.
Changed in version 1.4.0: Added the option to specify the filter as a callback.
- font_name¶
Filename of the font to use in UI components. The path can be absolute or relative. Relative paths are resolved by the
resource_find()
function.font_name
is aStringProperty
and defaults to ‘Roboto’. This value is taken fromConfig
.
- get_nice_size(fn)[source]¶
Pass the filepath. Returns the size in the best human readable format or ‘’ if it is a directory (Don’t recursively calculate size).
- layout¶
Reference to the layout widget instance.
layout is an
ObjectProperty
.New in version 1.9.0.
- multiselect¶
Determines whether the user is able to select multiple files or not.
multiselect is a
BooleanProperty
and defaults to False.
- on_touch_down(touch)[source]¶
Receive a touch down event.
- Parameters:
- touch:
MotionEvent
class Touch received. The touch is in parent coordinates. See
relativelayout
for a discussion on coordinate systems.
- touch:
- Returns:
bool If True, the dispatching of the touch event will stop. If False, the event will continue to be dispatched to the rest of the widget tree.
- on_touch_up(touch)[source]¶
Receive a touch up event. The touch is in parent coordinates.
See
on_touch_down()
for more information.
- path¶
path is a
StringProperty
and defaults to the current working directory as a unicode string. It specifies the path on the filesystem that this controller should refer to.Warning
If a unicode path is specified, all the files returned will be in unicode, allowing the display of unicode files and paths. If a bytes path is specified, only files and paths with ascii names will be displayed properly: non-ascii filenames will be displayed and listed with questions marks (?) instead of their unicode characters.
- progress_cls¶
Class to use for displaying a progress indicator for filechooser loading.
progress_cls is an
ObjectProperty
and defaults toFileChooserProgress
.New in version 1.2.0.
Changed in version 1.8.0: If set to a string, the
Factory
will be used to resolve the class name.
- rootpath¶
Root path to use instead of the system root path. If set, it will not show a “..” directory to go up to the root path. For example, if you set rootpath to /users/foo, the user will be unable to go to /users or to any other directory not starting with /users/foo.
rootpath is a
StringProperty
and defaults to None.New in version 1.2.0.
Note
Similarly to
path
, whether rootpath is specified as bytes or a unicode string determines the type of the filenames and paths read.
- selection¶
Contains the list of files that are currently selected.
selection is a read-only
ListProperty
and defaults to [].
Determines whether hidden files and folders should be shown.
show_hidden is a
BooleanProperty
and defaults to False.
- sort_func¶
Provides a function to be called with a list of filenames as the first argument and the filesystem implementation as the second argument. It returns a list of filenames sorted for display in the view.
sort_func is an
ObjectProperty
and defaults to a function returning alphanumerically named folders first.Changed in version 1.8.0: The signature needs now 2 arguments: first the list of files, second the filesystem class to use.
- class kivy.uix.filechooser.FileChooserIconLayout(**kwargs)[source]¶
Bases:
kivy.uix.filechooser.FileChooserLayout
File chooser layout using an icon view.
New in version 1.9.0.
- class kivy.uix.filechooser.FileChooserIconView(**kwargs)[source]¶
Bases:
kivy.uix.filechooser.FileChooserController
Implementation of a
FileChooserController
using an icon view.New in version 1.9.0.
- class kivy.uix.filechooser.FileChooserListLayout(**kwargs)[source]¶
Bases:
kivy.uix.filechooser.FileChooserLayout
File chooser layout using a list view.
New in version 1.9.0.
- class kivy.uix.filechooser.FileChooserListView(**kwargs)[source]¶
Bases:
kivy.uix.filechooser.FileChooserController
Implementation of a
FileChooserController
using a list view.New in version 1.9.0.
- class kivy.uix.filechooser.FileChooserProgressBase(**kwargs)[source]¶
Bases:
kivy.uix.floatlayout.FloatLayout
Base for implementing a progress view. This view is used when too many entries need to be created and are delayed over multiple frames.
New in version 1.2.0.
- on_touch_down(touch)[source]¶
Receive a touch down event.
- Parameters:
- touch:
MotionEvent
class Touch received. The touch is in parent coordinates. See
relativelayout
for a discussion on coordinate systems.
- touch:
- Returns:
bool If True, the dispatching of the touch event will stop. If False, the event will continue to be dispatched to the rest of the widget tree.
- on_touch_move(touch)[source]¶
Receive a touch move event. The touch is in parent coordinates.
See
on_touch_down()
for more information.
- on_touch_up(touch)[source]¶
Receive a touch up event. The touch is in parent coordinates.
See
on_touch_down()
for more information.
- path¶
Current path of the FileChooser, read-only.
- total¶
Total number of entries to load.
- class kivy.uix.filechooser.FileSystemAbstract[source]¶
Bases:
builtins.object
Class for implementing a File System view that can be used with the
FileChooser
.New in version 1.8.0.
Return True if the file is hidden
- class kivy.uix.filechooser.FileSystemLocal[source]¶
Bases:
kivy.uix.filechooser.FileSystemAbstract
Implementation of
FileSystemAbstract
for local files.New in version 1.8.0.
Return True if the file is hidden