Table Of Contents
- Label
- Sizing and text content
- Text alignment and wrapping
- Markup text
- Interactive zone in text
- Catering for Unicode languages
- Usage example
Label
Label.anchors
Label.base_direction
Label.bold
Label.color
Label.disabled_color
Label.disabled_outline_color
Label.ellipsis_options
Label.font_blended
Label.font_context
Label.font_direction
Label.font_family
Label.font_features
Label.font_hinting
Label.font_kerning
Label.font_name
Label.font_script_name
Label.font_size
Label.halign
Label.is_shortened
Label.italic
Label.limit_render_to_text_bbox
Label.line_height
Label.markup
Label.max_lines
Label.mipmap
Label.on_touch_down()
Label.outline_color
Label.outline_width
Label.padding
Label.padding_x
Label.padding_y
Label.refs
Label.shorten
Label.shorten_from
Label.split_str
Label.strikethrough
Label.strip
Label.text
Label.text_language
Label.text_size
Label.texture
Label.texture_size
Label.texture_update()
Label.underline
Label.unicode_errors
Label.valign
Label¶
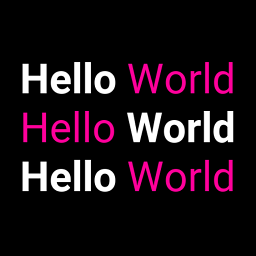
The Label
widget is for rendering text:
# hello world text
l = Label(text='Hello world')
# unicode text; can only display glyphs that are available in the font
l = Label(text='Hello world ' + chr(2764))
# multiline text
l = Label(text='Multi\nLine')
# size
l = Label(text='Hello world', font_size='20sp')
Sizing and text content¶
By default, the size of Label
is not affected by text
content and the text is not affected by the size. In order to control
sizing, you must specify text_size
to constrain the text
and/or bind size
to texture_size
to grow with
the text.
For example, this label’s size will be set to the text content
(plus padding
):
Label:
size: self.texture_size
This label’s text will wrap at the specified width and be clipped to the height:
Label:
text_size: cm(6), cm(4)
Combine these concepts to create a Label that can grow vertically but wraps the text at a certain width:
Label:
text_size: root.width, None
size: self.texture_size
How to have a custom background color in the label:
# Define your background color Template
<BackgroundColor@Widget>
background_color: 1, 1, 1, 1
canvas.before:
Color:
rgba: root.background_color
Rectangle:
size: self.size
pos: self.pos
# Now you can simply Mix the `BackgroundColor` class with almost
# any other widget... to give it a background.
<BackgroundLabel@Label+BackgroundColor>
background_color: 0, 0, 0, 0
# Default the background color for this label
# to r 0, g 0, b 0, a 0
# Use the BackgroundLabel any where in your kv code like below
BackgroundLabel
text: 'Hello'
background_color: 1, 0, 0, 1
Text alignment and wrapping¶
The Label
has halign
and valign
properties to control the alignment of its text. However, by default the text
image (texture
) is only just large enough to contain the
characters and is positioned in the center of the Label. The valign property
will have no effect and halign will only have an effect if your text has
newlines; a single line of text will appear to be centered even though halign
is set to left (by default).
In order for the alignment properties to take effect, set the
text_size
, which specifies the size of the bounding box within
which text is aligned. For instance, the following code binds this size to the
size of the Label, so text will be aligned within the widget bounds. This
will also automatically wrap the text of the Label to remain within this area.
Label:
text_size: self.size
halign: 'right'
valign: 'middle'
Markup text¶
New in version 1.1.0.
You can change the style of the text using Text Markup. The syntax is similar to the bbcode syntax but only the inline styling is allowed:
# hello world with world in bold
l = Label(text='Hello [b]World[/b]', markup=True)
# hello in red, world in blue
l = Label(text='[color=ff3333]Hello[/color][color=3333ff]World[/color]',
markup = True)
If you need to escape the markup from the current text, use
kivy.utils.escape_markup()
:
text = 'This is an important message [1]'
l = Label(text='[b]' + escape_markup(text) + '[/b]', markup=True)
The following tags are available:
[b][/b]
Activate bold text
[i][/i]
Activate italic text
[u][/u]
Underlined text
[s][/s]
Strikethrough text
[font=<str>][/font]
Change the font (note: this refers to a TTF file or registered alias)
[font_context=<str>][/font_context]
Change context for the font, use string value “none” for isolated context (this is equivalent to None; if you created a font context named ‘none’, it cannot be referred to using markup)
[font_family=<str>][/font_family]
Font family to request for drawing. This is only valid when using a font context, see
kivy.uix.label.Label
for details.[font_features=<str>][/font_features]
OpenType font features, in CSS format, this is passed straight through to Pango. The effects of requesting a feature depends on loaded fonts, library versions, etc. Pango only, requires v1.38 or later.
[size=<integer>][/size]
Change the font size
[color=#<color>][/color]
Change the text color
[ref=<str>][/ref]
Add an interactive zone. The reference + bounding box inside the reference will be available in
Label.refs
[anchor=<str>]
Put an anchor in the text. You can get the position of your anchor within the text with
Label.anchors
[sub][/sub]
Display the text at a subscript position relative to the text before it.
[sup][/sup]
Display the text at a superscript position relative to the text before it.
[text_language=<str>][/text_language]
Language of the text, this is an RFC-3066 format language tag (as string), for example “en_US”, “zh_CN”, “fr” or “ja”. This can impact font selection and metrics. Use the string “None” to revert to locale detection. Pango only.
If you want to render the markup text with a [ or ] or & character, you need to escape them. We created a simple syntax:
[ -> &bl;
] -> &br;
& -> &
Then you can write:
"[size=24]Hello &bl;World&br;[/size]"
Interactive zone in text¶
New in version 1.1.0.
You can now have definable “links” using text markup. The idea is to be able
to detect when the user clicks on part of the text and to react.
The tag [ref=xxx]
is used for that.
In this example, we are creating a reference on the word “World”. When
this word is clicked, the function print_it
will be called with the
name of the reference:
def print_it(instance, value):
print('User clicked on', value)
widget = Label(text='Hello [ref=world]World[/ref]', markup=True)
widget.bind(on_ref_press=print_it)
For prettier rendering, you could add a color for the reference. Replace the
text=
in the previous example with:
'Hello [ref=world][color=0000ff]World[/color][/ref]'
Catering for Unicode languages¶
The font kivy uses does not contain all the characters required for displaying all languages. When you use the built-in widgets, this results in a block being drawn where you expect a character.
If you want to display such characters, you can chose a font that supports them and deploy it universally via kv:
<Label>:
font_name: '/<path>/<to>/<font>'
Note that this needs to be done before your widgets are loaded as kv rules are only applied at load time.
Usage example¶
The following example marks the anchors and references contained in a label:
from kivy.app import App
from kivy.uix.label import Label
from kivy.clock import Clock
from kivy.graphics import Color, Rectangle
class TestApp(App):
@staticmethod
def get_x(label, ref_x):
""" Return the x value of the ref/anchor relative to the canvas """
return label.center_x - label.texture_size[0] * 0.5 + ref_x
@staticmethod
def get_y(label, ref_y):
""" Return the y value of the ref/anchor relative to the canvas """
# Note the inversion of direction, as y values start at the top of
# the texture and increase downwards
return label.center_y + label.texture_size[1] * 0.5 - ref_y
def show_marks(self, label):
# Indicate the position of the anchors with a red top marker
for name, anc in label.anchors.items():
with label.canvas:
Color(1, 0, 0)
Rectangle(pos=(self.get_x(label, anc[0]),
self.get_y(label, anc[1])),
size=(3, 3))
# Draw a green surround around the refs. Note the sizes y inversion
for name, boxes in label.refs.items():
for box in boxes:
with label.canvas:
Color(0, 1, 0, 0.25)
Rectangle(pos=(self.get_x(label, box[0]),
self.get_y(label, box[1])),
size=(box[2] - box[0],
box[1] - box[3]))
def build(self):
label = Label(
text='[anchor=a]a\nChars [anchor=b]b\n[ref=myref]ref[/ref]',
markup=True)
Clock.schedule_once(lambda dt: self.show_marks(label), 1)
return label
TestApp().run()
- class kivy.uix.label.Label(**kwargs)[source]¶
Bases:
kivy.uix.widget.Widget
Label class, see module documentation for more information.
- Events:
- on_ref_press
Fired when the user clicks on a word referenced with a
[ref]
tag in a text markup.
- anchors¶
New in version 1.1.0.
Position of all the
[anchor=xxx]
markup in the text. These coordinates are relative to the top left corner of the text, with the y value increasing downwards. Anchors names should be unique and only the first occurrence of any duplicate anchors will be recorded.You can place anchors in your markup text as follows:
text = """ [anchor=title1][size=24]This is my Big title.[/size] [anchor=content]Hello world """
Then, all the
[anchor=]
references will be removed and you’ll get all the anchor positions in this property (only after rendering):>>> widget = Label(text=text, markup=True) >>> widget.texture_update() >>> widget.anchors {"content": (20, 32), "title1": (20, 16)}
Note
This works only with markup text. You need
markup
set to True.
- base_direction¶
Base direction of text, this impacts horizontal alignment when
halign
is auto (the default). Available options are: None, “ltr” (left to right), “rtl” (right to left) plus “weak_ltr” and “weak_rtl”.Note
This feature requires the Pango text provider.
Note
Weak modes are currently not implemented in Kivy text layout, and have the same effect as setting strong mode.
New in version 1.11.0.
base_direction
is anOptionProperty
and defaults to None (autodetect RTL if possible, otherwise LTR).
- bold¶
Indicates use of the bold version of your font.
Note
Depending of your font, the bold attribute may have no impact on your text rendering.
bold
is aBooleanProperty
and defaults to False.
- color¶
Text color, in the format (r, g, b, a).
color
is aColorProperty
and defaults to [1, 1, 1, 1].Changed in version 2.0.0: Changed from
ListProperty
toColorProperty
.
- disabled_color¶
The color of the text when the widget is disabled, in the (r, g, b, a) format.
New in version 1.8.0.
disabled_color
is aColorProperty
and defaults to [1, 1, 1, .3].Changed in version 2.0.0: Changed from
ListProperty
toColorProperty
.
- disabled_outline_color¶
The color of the text outline when the widget is disabled, in the (r, g, b) format.
Note
This feature requires the SDL2 text provider.
New in version 1.10.0.
disabled_outline_color
is aColorProperty
and defaults to [0, 0, 0].Changed in version 2.0.0: Changed from
ListProperty
toColorProperty
. Alpha component is ignored and assigning value to it has no effect.
- ellipsis_options¶
Font options for the ellipsis string(’…’) used to split the text.
Accepts a dict as option name with the value. Only applied when
markup
is true and text is shortened. All font options which work forLabel
will work forellipsis_options
. Defaults for the options not specified are taken from the surronding text.Label: text: 'Some very long line which will be cut' markup: True shorten: True ellipsis_options: {'color':(1,0.5,0.5,1),'underline':True}
New in version 2.0.0.
ellipsis_options
is aDictProperty
and defaults to {} (the empty dict).
- font_blended¶
Whether blended or solid font rendering should be used.
Note
This feature requires the SDL2 text provider.
New in version 1.10.0.
font_blended
is aBooleanProperty
and defaults to True.
- font_context¶
Font context. None means the font is used in isolation, so you are guaranteed to be drawing with the TTF file resolved by
font_name
. Specifying a value here will load the font file into a named context, enabling fallback between all fonts in the same context. If a font context is set, you are not guaranteed that rendering will actually use the specified TTF file for all glyphs (Pango will pick the one it thinks is best).If Kivy is linked against a system-wide installation of FontConfig, you can load the system fonts by specifying a font context starting with the special string system://. This will load the system fontconfig configuration, and add your application-specific fonts on top of it (this imposes a significant risk of family name collision, Pango may not use your custom font file, but pick one from the system)
Note
This feature requires the Pango text provider.
New in version 1.11.0.
font_context
is aStringProperty
and defaults to None.
- font_direction¶
Direction for the specific font, can be one of ltr, rtl, ttb,`btt`.
font_direction currently only works with SDL2 ttf providers.
New in version 2.2.0.
font_direction
is aOptionProperty
and defults to ‘ltr’.
- font_family¶
Font family, this is only applicable when using
font_context
option. The specified font family will be requested, but note that it may not be available, or there could be multiple fonts registered with the same family. The value can be a family name (string) available in the font context (for example a system font in a system:// context, or a custom font file added usingkivy.core.text.FontContextManager
). If set to None, font selection is controlled by thefont_name
setting.Note
If using
font_name
to reference a custom font file, you should leave this as None. The family name is managed automatically in this case.Note
This feature requires the Pango text provider.
New in version 1.11.0.
font_family
is aStringProperty
and defaults to None.
- font_features¶
OpenType font features, in CSS format, this is passed straight through to Pango. The effects of requesting a feature depends on loaded fonts, library versions, etc. For a complete list of features, see:
https://en.wikipedia.org/wiki/List_of_typographic_features
Note
This feature requires the Pango text provider, and Pango library v1.38 or later.
New in version 1.11.0.
font_features
is aStringProperty
and defaults to an empty string.
- font_hinting¶
What hinting option to use for font rendering. Can be one of ‘normal’, ‘light’, ‘mono’ or None.
Note
This feature requires SDL2 or Pango text provider.
New in version 1.10.0.
font_hinting
is anOptionProperty
and defaults to ‘normal’.
- font_kerning¶
Whether kerning is enabled for font rendering. You should normally only disable this if rendering is broken with a particular font file.
Note
This feature requires the SDL2 text provider.
New in version 1.10.0.
font_kerning
is aBooleanProperty
and defaults to True.
- font_name¶
Filename of the font to use. The path can be absolute or relative. Relative paths are resolved by the
resource_find()
function.Warning
Depending of your text provider, the font file can be ignored. However, you can mostly use this without problems.
If the font used lacks the glyphs for the particular language/symbols you are using, you will see ‘[]’ blank box characters instead of the actual glyphs. The solution is to use a font that has the glyphs you need to display. For example, to display
, use a font such as freesans.ttf that has the glyph.
font_name
is aStringProperty
and defaults to ‘Roboto’. This value is taken fromConfig
.
- font_script_name¶
script_code from https://bit.ly/TypeScriptCodes .
New in version 2.2.0.
Warning
font_script_name is only currently supported in SDL2 ttf providers.
font_script_name
is aOptionProperty
and defaults to ‘Latn’.
- font_size¶
Font size of the text, in pixels.
font_size
is aNumericProperty
and defaults to 15sp.
- halign¶
Horizontal alignment of the text.
halign
is anOptionProperty
and defaults to ‘auto’. Available options are : auto, left, center, right and justify. Auto will attempt to autodetect horizontal alignment for RTL text (Pango only), otherwise it behaves like left.Warning
This doesn’t change the position of the text texture of the Label (centered), only the position of the text in this texture. You probably want to bind the size of the Label to the
texture_size
or set atext_size
.Changed in version 1.10.1: Added auto option
Changed in version 1.6.0: A new option was added to
halign
, namely justify.
- is_shortened¶
This property indicates if
text
was rendered with or without shortening whenshorten
is True.New in version 1.10.0.
is_shortened
is aBooleanProperty
and defaults to False.
- italic¶
Indicates use of the italic version of your font.
Note
Depending of your font, the italic attribute may have no impact on your text rendering.
italic
is aBooleanProperty
and defaults to False.
- limit_render_to_text_bbox¶
If set to
True
, this parameter indicates that rendering should be limited to the bounding box of the text, excluding any additional white spaces designated for ascent and descent.By limiting the rendering to the bounding box of the text, it ensures a more precise alignment with surrounding elements when utilizing properties such as valign, y, pos, pos_hint, etc.
Note
This feature requires the PIL text provider.
limit_render_to_text_bbox
is aBooleanProperty
and defaults to False.
- line_height¶
Line Height for the text. e.g. line_height = 2 will cause the spacing between lines to be twice the size.
line_height
is aNumericProperty
and defaults to 1.0.New in version 1.5.0.
- markup¶
New in version 1.1.0.
If True, the text will be rendered using the
MarkupLabel
: you can change the style of the text using tags. Check the Text Markup documentation for more information.markup
is aBooleanProperty
and defaults to False.
- max_lines¶
Maximum number of lines to use, defaults to 0, which means unlimited. Please note that
shorten
take over this property. (with shorten, the text is always one line.)New in version 1.8.0.
max_lines
is aNumericProperty
and defaults to 0.
- mipmap¶
Indicates whether OpenGL mipmapping is applied to the texture or not. Read Mipmapping for more information.
New in version 1.0.7.
mipmap
is aBooleanProperty
and defaults to False.
- on_touch_down(touch)[source]¶
Receive a touch down event.
- Parameters:
- touch:
MotionEvent
class Touch received. The touch is in parent coordinates. See
relativelayout
for a discussion on coordinate systems.
- touch:
- Returns:
bool If True, the dispatching of the touch event will stop. If False, the event will continue to be dispatched to the rest of the widget tree.
- outline_color¶
The color of the text outline, in the (r, g, b) format.
Note
This feature requires the SDL2 text provider.
New in version 1.10.0.
outline_color
is aColorProperty
and defaults to [0, 0, 0, 1].Changed in version 2.0.0: Changed from
ListProperty
toColorProperty
. Alpha component is ignored and assigning value to it has no effect.
- outline_width¶
Width in pixels for the outline around the text. No outline will be rendered if the value is None.
Note
This feature requires the SDL2 text provider.
New in version 1.10.0.
outline_width
is aNumericProperty
and defaults to None.
- padding¶
Padding of the text in the format [padding_left, padding_top, padding_right, padding_bottom]
padding
also accepts a two argument form [padding_horizontal, padding_vertical] and a one argument form [padding].Changed in version 2.2.0: Replaced ReferenceListProperty with VariableListProperty.
padding
is aVariableListProperty
and defaults to [0, 0, 0, 0].
- padding_x¶
Horizontal padding of the text inside the widget box.
padding_x
is aNumericProperty
and defaults to 0.Changed in version 1.9.0: padding_x has been fixed to work as expected. In the past, the text was padded by the negative of its values.
Deprecated since version 2.2.0: Please use
padding
instead.
- padding_y¶
Vertical padding of the text inside the widget box.
padding_y
is aNumericProperty
and defaults to 0.Changed in version 1.9.0: padding_y has been fixed to work as expected. In the past, the text was padded by the negative of its values.
Deprecated since version 2.2.0: Please use
padding
instead.
- refs¶
New in version 1.1.0.
List of
[ref=xxx]
markup items in the text with the bounding box of all the words contained in a ref, available only after rendering.For example, if you wrote:
Check out my [ref=hello]link[/ref]
The refs will be set with:
{'hello': ((64, 0, 78, 16), )}
The references marked “hello” have a bounding box at (x1, y1, x2, y2). These coordinates are relative to the top left corner of the text, with the y value increasing downwards. You can define multiple refs with the same name: each occurrence will be added as another (x1, y1, x2, y2) tuple to this list.
The current Label implementation uses these references if they exist in your markup text, automatically doing the collision with the touch and dispatching an on_ref_press event.
You can bind a ref event like this:
def print_it(instance, value): print('User click on', value) widget = Label(text='Hello [ref=world]World[/ref]', markup=True) widget.bind(on_ref_press=print_it)
Note
This works only with markup text. You need
markup
set to True.
- shorten¶
Indicates whether the label should attempt to shorten its textual contents as much as possible if a
text_size
is given. Setting this to True without an appropriately settext_size
will lead to unexpected results.shorten_from
andsplit_str
control the direction from which thetext
is split, as well as where in thetext
we are allowed to split.shorten
is aBooleanProperty
and defaults to False.
- shorten_from¶
The side from which we should shorten the text from, can be left, right, or center.
For example, if left, the ellipsis will appear towards the left side and we will display as much text starting from the right as possible. Similar to
shorten
, this option only applies whentext_size
[0] is not None, In this case, the string is shortened to fit within the specified width.New in version 1.9.0.
shorten_from
is aOptionProperty
and defaults to center.
- split_str¶
The string used to split the
text
while shortening the string whenshorten
is True.For example, if it’s a space, the string will be broken into words and as many whole words that can fit into a single line will be displayed. If
split_str
is the empty string, ‘’, we split on every character fitting as much text as possible into the line.New in version 1.9.0.
split_str
is aStringProperty
and defaults to ‘’ (the empty string).
- strikethrough¶
Adds a strikethrough line to the text.
Note
This feature requires the SDL2 text provider.
New in version 1.10.0.
strikethrough
is aBooleanProperty
and defaults to False.
- strip¶
Whether leading and trailing spaces and newlines should be stripped from each displayed line. If True, every line will start at the right or left edge, depending on
halign
. Ifhalign
is justify it is implicitly True.New in version 1.9.0.
strip
is aBooleanProperty
and defaults to False.
- text¶
Text of the label.
Creation of a simple hello world:
widget = Label(text='Hello world')
text
is aStringProperty
and defaults to ‘’.
- text_language¶
Language of the text, if None Pango will determine it from locale. This is an RFC-3066 format language tag (as a string), for example “en_US”, “zh_CN”, “fr” or “ja”. This can impact font selection, metrics and rendering. For example, the same bytes of text can look different for ur and ar languages, though both use Arabic script.
Note
This feature requires the Pango text provider.
New in version 1.11.0.
text_language
is aStringProperty
and defaults to None.
- text_size¶
By default, the label is not constrained to any bounding box. You can set the size constraint of the label with this property. The text will autoflow into the constraints. So although the font size will not be reduced, the text will be arranged to fit into the box as best as possible, with any text still outside the box clipped.
This sets and clips
texture_size
to text_size if not None.New in version 1.0.4.
For example, whatever your current widget size is, if you want the label to be created in a box with width=200 and unlimited height:
Label(text='Very big big line', text_size=(200, None))
Note
This text_size property is the same as the
usersize
property in theLabel
class. (It is named size= in the constructor.)text_size
is aListProperty
and defaults to (None, None), meaning no size restriction by default.
- texture¶
Texture object of the text. The text is rendered automatically when a property changes. The OpenGL texture created in this operation is stored in this property. You can use this
texture
for any graphics elements.Depending on the texture creation, the value will be a
Texture
orTextureRegion
object.Warning
The
texture
update is scheduled for the next frame. If you need the texture immediately after changing a property, you have to call thetexture_update()
method before accessingtexture
:l = Label(text='Hello world') # l.texture is good l.font_size = '50sp' # l.texture is not updated yet l.texture_update() # l.texture is good now.
texture
is anObjectProperty
and defaults to None.
- texture_size¶
Texture size of the text. The size is determined by the font size and text. If
text_size
is [None, None], the texture will be the size required to fit the text, otherwise it’s clipped to fittext_size
.When
text_size
is [None, None], one can bind to texture_size and rescale it proportionally to fit the size of the label in order to make the text fit maximally in the label.Warning
The
texture_size
is set after thetexture
property. If you listen for changes totexture
,texture_size
will not be up-to-date in your callback. Bind totexture_size
instead.
- texture_update(*largs)[source]¶
Force texture recreation with the current Label properties.
After this function call, the
texture
andtexture_size
will be updated in this order.
- underline¶
Adds an underline to the text.
Note
This feature requires the SDL2 text provider.
New in version 1.10.0.
underline
is aBooleanProperty
and defaults to False.
- unicode_errors¶
How to handle unicode decode errors. Can be ‘strict’, ‘replace’ or ‘ignore’.
New in version 1.9.0.
unicode_errors
is anOptionProperty
and defaults to ‘replace’.
- valign¶
Vertical alignment of the text.
valign
is anOptionProperty
and defaults to ‘bottom’. Available options are : ‘bottom’, ‘middle’ (or ‘center’) and ‘top’.Changed in version 1.10.0: The ‘center’ option has been added as an alias of ‘middle’.
Warning
This doesn’t change the position of the text texture of the Label (centered), only the position of the text within this texture. You probably want to bind the size of the Label to the
texture_size
or set atext_size
to change this behavior.