Table Of Contents
TabbedPanel¶
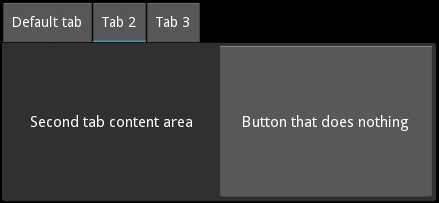
New in version 1.3.0.
The TabbedPanel widget manages different widgets in tabs, with a header area for the actual tab buttons and a content area for showing the current tab content.
The TabbedPanel
provides one default tab.
Simple example¶
'''
TabbedPanel
============
Test of the widget TabbedPanel.
'''
from kivy.app import App
from kivy.uix.tabbedpanel import TabbedPanel
from kivy.lang import Builder
Builder.load_string("""
<Test>:
size_hint: .5, .5
pos_hint: {'center_x': .5, 'center_y': .5}
do_default_tab: False
TabbedPanelItem:
text: 'first tab'
Label:
text: 'First tab content area'
TabbedPanelItem:
text: 'tab2'
BoxLayout:
Label:
text: 'Second tab content area'
Button:
text: 'Button that does nothing'
TabbedPanelItem:
text: 'tab3'
RstDocument:
text:
'\\n'.join(("Hello world", "-----------",
"You are in the third tab."))
""")
class Test(TabbedPanel):
pass
class TabbedPanelApp(App):
def build(self):
return Test()
if __name__ == '__main__':
TabbedPanelApp().run()
Note
A new class TabbedPanelItem
has been introduced in 1.5.0 for
convenience. So now one can simply add a TabbedPanelItem
to a
TabbedPanel
and content to the TabbedPanelItem
as in the example provided above.
Customize the Tabbed Panel¶
You can choose the position in which the tabs are displayed:
tab_pos = 'top_mid'
An individual tab is called a TabbedPanelHeader. It is a special button containing a content property. You add the TabbedPanelHeader first, and set its content property separately:
tp = TabbedPanel()
th = TabbedPanelHeader(text='Tab2')
tp.add_widget(th)
An individual tab, represented by a TabbedPanelHeader, needs its content set. This content can be any widget. It could be a layout with a deep hierarchy of widgets, or it could be an individual widget, such as a label or a button:
th.content = your_content_instance
There is one “shared” main content area active at any given time, for all the tabs. Your app is responsible for adding the content of individual tabs and for managing them, but it’s not responsible for content switching. The tabbed panel handles switching of the main content object as per user action.
There is a default tab added when the tabbed panel is instantiated. Tabs that you add individually as above, are added in addition to the default tab. Thus, depending on your needs and design, you will want to customize the default tab:
tp.default_tab_text = 'Something Specific To Your Use'
The default tab machinery requires special consideration and management. Accordingly, an on_default_tab event is provided for associating a callback:
tp.bind(default_tab = my_default_tab_callback)
It’s important to note that by default, default_tab_cls
is of type
TabbedPanelHeader
and thus has the same properties as other tabs.
Since 1.5.0, it is now possible to disable the creation of the
default_tab
by setting do_default_tab
to False.
Tabs and content can be removed in several ways:
tp.remove_widget(widget/tabbed_panel_header)
or
tp.clear_widgets() # to clear all the widgets in the content area
or
tp.clear_tabs() # to remove the TabbedPanelHeaders
To access the children of the tabbed panel, use content.children:
tp.content.children
To access the list of tabs:
tp.tab_list
To change the appearance of the main tabbed panel content:
background_color = (1, 0, 0, .5) #50% translucent red
border = [0, 0, 0, 0]
background_image = 'path/to/background/image'
To change the background of a individual tab, use these two properties:
tab_header_instance.background_normal = 'path/to/tab_head/img'
tab_header_instance.background_down = 'path/to/tab_head/img_pressed'
A TabbedPanelStrip contains the individual tab headers. To change the appearance of this tab strip, override the canvas of TabbedPanelStrip. For example, in the kv language:
<TabbedPanelStrip>
canvas:
Color:
rgba: (0, 1, 0, 1) # green
Rectangle:
size: self.size
pos: self.pos
By default the tabbed panel strip takes its background image and color from the tabbed panel’s background_image and background_color.
-
class
kivy.uix.tabbedpanel.
StripLayout
(**kwargs)[source]¶ Bases:
kivy.uix.gridlayout.GridLayout
The main layout that is used to house the entire tabbedpanel strip including the blank areas in case the tabs don’t cover the entire width/height.
New in version 1.8.0.
-
background_image
¶ Background image to be used for the Strip layout of the TabbedPanel.
background_image
is aStringProperty
and defaults to a transparent image.
-
border
¶ Border property for the
background_image
.border
is aListProperty
and defaults to [4, 4, 4, 4]
-
-
class
kivy.uix.tabbedpanel.
TabbedPanel
(**kwargs)[source]¶ Bases:
kivy.uix.gridlayout.GridLayout
The TabbedPanel class. See module documentation for more information.
-
add_widget
(widget, index=0)[source]¶ Add a new widget as a child of this widget.
Parameters: - widget:
Widget
Widget to add to our list of children.
- index: int, defaults to 0
Index to insert the widget in the list. Notice that the default of 0 means the widget is inserted at the beginning of the list and will thus be drawn on top of other sibling widgets. For a full discussion of the index and widget hierarchy, please see the Widgets Programming Guide.
New in version 1.0.5.
- canvas: str, defaults to None
Canvas to add widget’s canvas to. Can be ‘before’, ‘after’ or None for the default canvas.
New in version 1.9.0.
>>> from kivy.uix.button import Button >>> from kivy.uix.slider import Slider >>> root = Widget() >>> root.add_widget(Button()) >>> slider = Slider() >>> root.add_widget(slider)
- widget:
-
background_color
¶ Background color, in the format (r, g, b, a).
background_color
is aListProperty
and defaults to [1, 1, 1, 1].
-
background_disabled_image
¶ Background image of the main shared content object when disabled.
New in version 1.8.0.
background_disabled_image
is aStringProperty
and defaults to ‘atlas://data/images/defaulttheme/tab’.
-
background_image
¶ Background image of the main shared content object.
background_image
is aStringProperty
and defaults to ‘atlas://data/images/defaulttheme/tab’.
-
border
¶ Border used for
BorderImage
graphics instruction, used itself forbackground_image
. Can be changed for a custom background.It must be a list of four values: (bottom, right, top, left). Read the BorderImage instructions for more information.
border
is aListProperty
and defaults to (16, 16, 16, 16)
-
clear_widgets
(**kwargs)[source]¶ Remove all (or the specified)
children
of this widget. If the ‘children’ argument is specified, it should be a list (or filtered list) of children of the current widget.Changed in version 1.8.0: The children argument can be used to specify the children you want to remove.
-
content
¶ This is the object holding (current_tab’s content is added to this) the content of the current tab. To Listen to the changes in the content of the current tab, you should bind to current_tabs content property.
content
is anObjectProperty
and defaults to ‘None’.
-
current_tab
¶ Links to the currently selected or active tab.
New in version 1.4.0.
current_tab
is anAliasProperty
, read-only.
-
default_tab
¶ Holds the default tab.
Note
For convenience, the automatically provided default tab is deleted when you change default_tab to something else. As of 1.5.0, this behaviour has been extended to every default_tab for consistency and not just the automatically provided one.
default_tab
is anAliasProperty
.
-
default_tab_cls
¶ Specifies the class to use for the styling of the default tab.
New in version 1.4.0.
Warning
default_tab_cls should be subclassed from TabbedPanelHeader
default_tab_cls
is anObjectProperty
and defaults to TabbedPanelHeader. If you set a string, theFactory
will be used to resolve the class.Changed in version 1.8.0: The
Factory
will resolve the class if a string is set.
-
default_tab_content
¶ Holds the default tab content.
default_tab_content
is anAliasProperty
.
-
default_tab_text
¶ Specifies the text displayed on the default tab header.
default_tab_text
is aStringProperty
and defaults to ‘default tab’.
-
do_default_tab
¶ Specifies whether a default_tab head is provided.
New in version 1.5.0.
do_default_tab
is aBooleanProperty
and defaults to ‘True’.
-
remove_widget
(widget)[source]¶ Remove a widget from the children of this widget.
Parameters: - widget:
Widget
Widget to remove from our children list.
>>> from kivy.uix.button import Button >>> root = Widget() >>> button = Button() >>> root.add_widget(button) >>> root.remove_widget(button)
- widget:
-
strip_border
¶ Border to be used on
strip_image
.New in version 1.8.0.
strip_border
is aListProperty
and defaults to [4, 4, 4, 4].
-
strip_image
¶ Background image of the tabbed strip.
New in version 1.8.0.
strip_image
is aStringProperty
and defaults to a empty image.
-
switch_to
(header, do_scroll=False)[source]¶ Switch to a specific panel header.
Changed in version 1.10.0.
If used with do_scroll=True, it scrolls to the header’s tab too.
-
tab_height
¶ Specifies the height of the tab header.
tab_height
is aNumericProperty
and defaults to 40.
-
tab_list
¶ List of all the tab headers.
tab_list
is anAliasProperty
and is read-only.
-
tab_pos
¶ Specifies the position of the tabs relative to the content. Can be one of: left_top, left_mid, left_bottom, top_left, top_mid, top_right, right_top, right_mid, right_bottom, bottom_left, bottom_mid, bottom_right.
tab_pos
is anOptionProperty
and defaults to ‘top_left’.
-
tab_width
¶ Specifies the width of the tab header.
tab_width
is aNumericProperty
and defaults to 100.
-
-
class
kivy.uix.tabbedpanel.
TabbedPanelContent
(**kwargs)[source]¶ Bases:
kivy.uix.floatlayout.FloatLayout
The TabbedPanelContent class.
-
class
kivy.uix.tabbedpanel.
TabbedPanelHeader
(**kwargs)[source]¶ Bases:
kivy.uix.togglebutton.ToggleButton
A Base for implementing a Tabbed Panel Head. A button intended to be used as a Heading/Tab for a TabbedPanel widget.
You can use this TabbedPanelHeader widget to add a new tab to a TabbedPanel.
-
content
¶ Content to be loaded when this tab header is selected.
content
is anObjectProperty
and defaults to None.
-
on_touch_down
(touch)[source]¶ Receive a touch down event.
Parameters: - touch:
MotionEvent
class Touch received. The touch is in parent coordinates. See
relativelayout
for a discussion on coordinate systems.
Returns: bool If True, the dispatching of the touch event will stop. If False, the event will continue to be dispatched to the rest of the widget tree.
- touch:
-
-
class
kivy.uix.tabbedpanel.
TabbedPanelItem
(**kwargs)[source]¶ Bases:
kivy.uix.tabbedpanel.TabbedPanelHeader
This is a convenience class that provides a header of type TabbedPanelHeader and links it with the content automatically. Thus facilitating you to simply do the following in kv language:
<TabbedPanel>: # ...other settings TabbedPanelItem: BoxLayout: Label: text: 'Second tab content area' Button: text: 'Button that does nothing'
New in version 1.5.0.
-
add_widget
(widget, index=0)[source]¶ Add a new widget as a child of this widget.
Parameters: - widget:
Widget
Widget to add to our list of children.
- index: int, defaults to 0
Index to insert the widget in the list. Notice that the default of 0 means the widget is inserted at the beginning of the list and will thus be drawn on top of other sibling widgets. For a full discussion of the index and widget hierarchy, please see the Widgets Programming Guide.
New in version 1.0.5.
- canvas: str, defaults to None
Canvas to add widget’s canvas to. Can be ‘before’, ‘after’ or None for the default canvas.
New in version 1.9.0.
>>> from kivy.uix.button import Button >>> from kivy.uix.slider import Slider >>> root = Widget() >>> root.add_widget(Button()) >>> slider = Slider() >>> root.add_widget(slider)
- widget:
-
-
class
kivy.uix.tabbedpanel.
TabbedPanelStrip
(**kwargs)[source]¶ Bases:
kivy.uix.gridlayout.GridLayout
A strip intended to be used as background for Heading/Tab. This does not cover the blank areas in case the tabs don’t cover the entire width/height of the TabbedPanel(use
StripLayout
for that).-
tabbed_panel
¶ Link to the panel that the tab strip is a part of.
tabbed_panel
is anObjectProperty
and defaults to None .
-