Table Of Contents
Splitter¶
New in version 1.5.0.
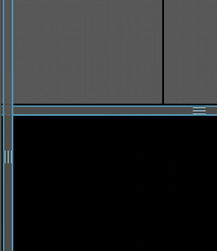
The Splitter
is a widget that helps you re-size its child
widget/layout by letting you re-size it via dragging the boundary or
double tapping the boundary. This widget is similar to the
ScrollView
in that it allows only one
child widget.
Usage:
splitter = Splitter(sizable_from = 'right')
splitter.add_widget(layout_or_widget_instance)
splitter.min_size = 100
splitter.max_size = 250
To change the size of the strip/border used for resizing:
splitter.strip_size = '10pt'
To change its appearance:
splitter.strip_cls = your_custom_class
You can also change the appearance of the strip_cls, which defaults to
SplitterStrip
, by overriding the kv rule in your app:
<SplitterStrip>:
horizontal: True if self.parent and self.parent.sizable_from[0] in ('t', 'b') else False
background_normal: 'path to normal horizontal image' if self.horizontal else 'path to vertical normal image'
background_down: 'path to pressed horizontal image' if self.horizontal else 'path to vertical pressed image'
-
class
kivy.uix.splitter.
Splitter
(**kwargs)[source]¶ Bases:
kivy.uix.boxlayout.BoxLayout
See module documentation.
Events: - on_press:
Fired when the splitter is pressed.
- on_release:
Fired when the splitter is released.
Changed in version 1.6.0: Added on_press and on_release events.
-
add_widget
(widget, index=0)[source]¶ Add a new widget as a child of this widget.
Parameters: - widget:
Widget
Widget to add to our list of children.
- index: int, defaults to 0
Index to insert the widget in the list. Notice that the default of 0 means the widget is inserted at the beginning of the list and will thus be drawn on top of other sibling widgets. For a full discussion of the index and widget hierarchy, please see the Widgets Programming Guide.
New in version 1.0.5.
- canvas: str, defaults to None
Canvas to add widget’s canvas to. Can be ‘before’, ‘after’ or None for the default canvas.
New in version 1.9.0.
>>> from kivy.uix.button import Button >>> from kivy.uix.slider import Slider >>> root = Widget() >>> root.add_widget(Button()) >>> slider = Slider() >>> root.add_widget(slider)
- widget:
-
border
¶ Border used for the
BorderImage
graphics instruction.This must be a list of four values: (bottom, right, top, left). Read the BorderImage instructions for more information about how to use it.
border
is aListProperty
and defaults to (4, 4, 4, 4).
-
clear_widgets
()[source]¶ Remove all (or the specified)
children
of this widget. If the ‘children’ argument is specified, it should be a list (or filtered list) of children of the current widget.Changed in version 1.8.0: The children argument can be used to specify the children you want to remove.
-
keep_within_parent
¶ If True, will limit the splitter to stay within its parent widget.
keep_within_parent
is aBooleanProperty
and defaults to False.New in version 1.9.0.
-
max_size
¶ Specifies the maximum size beyond which the widget is not resizable.
max_size
is aNumericProperty
and defaults to 500pt.
-
min_size
¶ Specifies the minimum size beyond which the widget is not resizable.
min_size
is aNumericProperty
and defaults to 100pt.
-
remove_widget
(widget, *largs)[source]¶ Remove a widget from the children of this widget.
Parameters: - widget:
Widget
Widget to remove from our children list.
>>> from kivy.uix.button import Button >>> root = Widget() >>> button = Button() >>> root.add_widget(button) >>> root.remove_widget(button)
- widget:
-
rescale_with_parent
¶ If True, will automatically change size to take up the same proportion of the parent widget when it is resized, while staying within
min_size
andmax_size
. As long as these attributes can be satisfied, this stops theSplitter
from exceeding the parent size during rescaling.rescale_with_parent
is aBooleanProperty
and defaults to False.New in version 1.9.0.
-
sizable_from
¶ Specifies whether the widget is resizable. Options are: left, right, top or bottom
sizable_from
is anOptionProperty
and defaults to left.
-
strip_cls
¶ Specifies the class of the resize Strip.
strip_cls
is ankivy.properties.ObjectProperty
and defaults toSplitterStrip
, which is of typeButton
.Changed in version 1.8.0: If you set a string, the
Factory
will be used to resolve the class.
-
strip_size
¶ Specifies the size of resize strip
strp_size
is aNumericProperty
defaults to 10pt