Table Of Contents
Color Picker¶
New in version 1.7.0.
Warning
This widget is experimental. Its use and API can change at any time until this warning is removed.
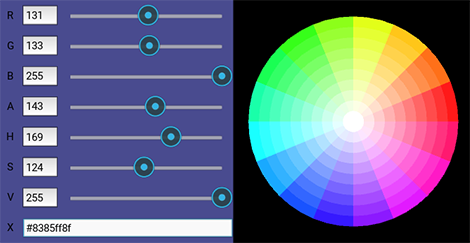
The ColorPicker widget allows a user to select a color from a chromatic wheel where pinch and zoom can be used to change the wheel’s saturation. Sliders and TextInputs are also provided for entering the RGBA/HSV/HEX values directly.
Usage:
clr_picker = ColorPicker()
parent.add_widget(clr_picker)
# To monitor changes, we can bind to color property changes
def on_color(instance, value):
print("RGBA = ", str(value)) # or instance.color
print("HSV = ", str(instance.hsv))
print("HEX = ", str(instance.hex_color))
clr_picker.bind(color=on_color)
- class kivy.uix.colorpicker.ColorPicker(**kwargs)[source]¶
Bases:
kivy.uix.relativelayout.RelativeLayout
See module documentation.
- color¶
The
color
holds the color currently selected in rgba format.color
is aListProperty
and defaults to (1, 1, 1, 1).
- font_name¶
Specifies the font used on the ColorPicker.
font_name
is aStringProperty
and defaults to ‘data/fonts/RobotoMono-Regular.ttf’.
- hex_color¶
The
hex_color
holds the currently selected color in hex.hex_color
is anAliasProperty
and defaults to #ffffffff.
- hsv¶
The
hsv
holds the color currently selected in hsv format.hsv
is aListProperty
and defaults to (1, 1, 1).
- wheel¶
The
wheel
holds the color wheel.wheel
is anObjectProperty
and defaults to None.
- class kivy.uix.colorpicker.ColorWheel(**kwargs)[source]¶
Bases:
kivy.uix.widget.Widget
Chromatic wheel for the ColorPicker.
Changed in version 1.7.1: font_size, font_name and foreground_color have been removed. The sizing is now the same as others widget, based on ‘sp’. Orientation is also automatically determined according to the width/height ratio.
- a¶
The Alpha value of the color currently selected.
a
is aBoundedNumericProperty
and can be a value from 0 to 1. It defaults to 0.
- b¶
The Blue value of the color currently selected.
b
is aBoundedNumericProperty
and can be a value from 0 to 1. It defaults to 0.
- color¶
The holds the color currently selected.
color
is aReferenceListProperty
and contains a list of r, g, b, a values. It defaults to [0, 0, 0, 0].
- g¶
The Green value of the color currently selected.
g
is aBoundedNumericProperty
and can be a value from 0 to 1. It defaults to 0.
- on_touch_down(touch)[source]¶
Receive a touch down event.
- Parameters:
- touch:
MotionEvent
class Touch received. The touch is in parent coordinates. See
relativelayout
for a discussion on coordinate systems.
- touch:
- Returns:
bool If True, the dispatching of the touch event will stop. If False, the event will continue to be dispatched to the rest of the widget tree.
- on_touch_move(touch)[source]¶
Receive a touch move event. The touch is in parent coordinates.
See
on_touch_down()
for more information.
- on_touch_up(touch)[source]¶
Receive a touch up event. The touch is in parent coordinates.
See
on_touch_down()
for more information.
- r¶
The Red value of the color currently selected.
r
is aBoundedNumericProperty
and can be a value from 0 to 1. It defaults to 0.