Table Of Contents
- Screen Manager
- Basic Usage
- Changing Direction
- Advanced Usage
- Changing transitions
CardTransition
FadeTransition
FallOutTransition
NoTransition
RiseInTransition
Screen
ScreenManager
ScreenManager.add_widget()
ScreenManager.clear_widgets()
ScreenManager.current
ScreenManager.current_screen
ScreenManager.get_screen()
ScreenManager.has_screen()
ScreenManager.next()
ScreenManager.on_motion()
ScreenManager.on_touch_down()
ScreenManager.on_touch_move()
ScreenManager.on_touch_up()
ScreenManager.previous()
ScreenManager.remove_widget()
ScreenManager.screen_names
ScreenManager.screens
ScreenManager.switch_to()
ScreenManager.transition
ScreenManagerException
ShaderTransition
SlideTransition
SwapTransition
TransitionBase
WipeTransition
Screen Manager¶
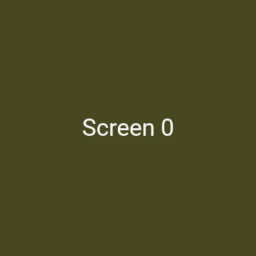
New in version 1.4.0.
The screen manager is a widget dedicated to managing multiple screens for your
application. The default ScreenManager
displays only one
Screen
at a time and uses a TransitionBase
to switch from one
Screen to another.
Multiple transitions are supported based on changing the screen coordinates / scale or even performing fancy animation using custom shaders.
Basic Usage¶
Let’s construct a Screen Manager with 4 named screens. When you are creating a screen, you absolutely need to give a name to it:
from kivy.uix.screenmanager import ScreenManager, Screen
# Create the manager
sm = ScreenManager()
# Add few screens
for i in range(4):
screen = Screen(name='Title %d' % i)
sm.add_widget(screen)
# By default, the first screen added into the ScreenManager will be
# displayed. You can then change to another screen.
# Let's display the screen named 'Title 2'
# A transition will automatically be used.
sm.current = 'Title 2'
The default ScreenManager.transition
is a SlideTransition
with
options direction
and
duration
.
Please note that by default, a Screen
displays nothing: it’s just a
RelativeLayout
. You need to use that class as
a root widget for your own screen, the best way being to subclass.
Warning
As Screen
is a RelativeLayout
,
it is important to understand the
Common Pitfalls.
Here is an example with a ‘Menu Screen’ and a ‘Settings Screen’:
from kivy.app import App
from kivy.lang import Builder
from kivy.uix.screenmanager import ScreenManager, Screen
# Create both screens. Please note the root.manager.current: this is how
# you can control the ScreenManager from kv. Each screen has by default a
# property manager that gives you the instance of the ScreenManager used.
Builder.load_string("""
<MenuScreen>:
BoxLayout:
Button:
text: 'Goto settings'
on_press: root.manager.current = 'settings'
Button:
text: 'Quit'
<SettingsScreen>:
BoxLayout:
Button:
text: 'My settings button'
Button:
text: 'Back to menu'
on_press: root.manager.current = 'menu'
""")
# Declare both screens
class MenuScreen(Screen):
pass
class SettingsScreen(Screen):
pass
class TestApp(App):
def build(self):
# Create the screen manager
sm = ScreenManager()
sm.add_widget(MenuScreen(name='menu'))
sm.add_widget(SettingsScreen(name='settings'))
return sm
if __name__ == '__main__':
TestApp().run()
Changing Direction¶
A common use case for ScreenManager
involves using a
SlideTransition
which slides right to the next screen
and slides left to the previous screen. Building on the previous
example, this can be accomplished like so:
Builder.load_string("""
<MenuScreen>:
BoxLayout:
Button:
text: 'Goto settings'
on_press:
root.manager.transition.direction = 'left'
root.manager.current = 'settings'
Button:
text: 'Quit'
<SettingsScreen>:
BoxLayout:
Button:
text: 'My settings button'
Button:
text: 'Back to menu'
on_press:
root.manager.transition.direction = 'right'
root.manager.current = 'menu'
""")
Advanced Usage¶
From 1.8.0, you can now switch dynamically to a new screen, change the
transition options and remove the previous one by using
switch_to()
:
sm = ScreenManager()
screens = [Screen(name='Title {}'.format(i)) for i in range(4)]
sm.switch_to(screens[0])
# later
sm.switch_to(screens[1], direction='right')
Note that this method adds the screen to the ScreenManager
instance
and should not be used if your screens have already been added to this
instance. To switch to a screen which is already added, you should use the
current
property.
Changing transitions¶
You have multiple transitions available by default, such as:
NoTransition
- switches screens instantly with no animationSlideTransition
- slide the screen in/out, from any directionCardTransition
- new screen slides on the previous or the old one slides off the new one depending on the modeSwapTransition
- implementation of the iOS swap transitionFadeTransition
- shader to fade the screen in/outWipeTransition
- shader to wipe the screens from right to leftFallOutTransition
- shader where the old screen ‘falls’ and becomes transparent, revealing the new one behind it.RiseInTransition
- shader where the new screen rises from the screen centre while fading from transparent to opaque.
You can easily switch transitions by changing the
ScreenManager.transition
property:
sm = ScreenManager(transition=FadeTransition())
Note
Currently, none of Shader based Transitions use anti-aliasing. This is because they use the FBO which doesn’t have any logic to handle supersampling. This is a known issue and we are working on a transparent implementation that will give the same results as if it had been rendered on screen.
To be more concrete, if you see sharp edged text during the animation, it’s normal.
- class kivy.uix.screenmanager.CardTransition[source]¶
Bases:
kivy.uix.screenmanager.SlideTransition
Card transition that looks similar to Android 4.x application drawer interface animation.
It supports 4 directions like SlideTransition: left, right, up and down, and two modes, pop and push. If push mode is activated, the previous screen does not move, and the new one slides in from the given direction. If the pop mode is activated, the previous screen slides out, when the new screen is already on the position of the ScreenManager.
New in version 1.10.
- mode¶
Indicates if the transition should push or pop the screen on/off the ScreenManager.
‘push’ means the screen slides in in the given direction
‘pop’ means the screen slides out in the given direction
mode
is anOptionProperty
and defaults to ‘push’.
- start(manager)[source]¶
(internal) Starts the transition. This is automatically called by the
ScreenManager
.
- class kivy.uix.screenmanager.FadeTransition[source]¶
Bases:
kivy.uix.screenmanager.ShaderTransition
Fade transition, based on a fragment Shader.
- fs¶
Fragment shader to use.
fs
is aStringProperty
and defaults to None.
- class kivy.uix.screenmanager.FallOutTransition[source]¶
Bases:
kivy.uix.screenmanager.ShaderTransition
Transition where the new screen ‘falls’ from the screen centre, becoming smaller and more transparent until it disappears, and revealing the new screen behind it. Mimics the popular/standard Android transition.
New in version 1.8.0.
- duration¶
Duration in seconds of the transition, replacing the default of
TransitionBase
.duration
is aNumericProperty
and defaults to .15 (= 150ms).
- fs¶
Fragment shader to use.
fs
is aStringProperty
and defaults to None.
- class kivy.uix.screenmanager.NoTransition[source]¶
Bases:
kivy.uix.screenmanager.TransitionBase
No transition, instantly switches to the next screen with no delay or animation.
New in version 1.8.0.
- duration¶
Duration in seconds of the transition.
duration
is aNumericProperty
and defaults to .4 (= 400ms).Changed in version 1.8.0: Default duration has been changed from 700ms to 400ms.
- class kivy.uix.screenmanager.RiseInTransition[source]¶
Bases:
kivy.uix.screenmanager.ShaderTransition
Transition where the new screen rises from the screen centre, becoming larger and changing from transparent to opaque until it fills the screen. Mimics the popular/standard Android transition.
New in version 1.8.0.
- duration¶
Duration in seconds of the transition, replacing the default of
TransitionBase
.duration
is aNumericProperty
and defaults to .2 (= 200ms).
- fs¶
Fragment shader to use.
fs
is aStringProperty
and defaults to None.
- class kivy.uix.screenmanager.Screen(**kw)[source]¶
Bases:
kivy.uix.relativelayout.RelativeLayout
Screen is an element intended to be used with a
ScreenManager
. Check module documentation for more information.- Events:
- on_pre_enter: ()
Event fired when the screen is about to be used: the entering animation is started.
- on_enter: ()
Event fired when the screen is displayed: the entering animation is complete.
- on_pre_leave: ()
Event fired when the screen is about to be removed: the leaving animation is started.
- on_leave: ()
Event fired when the screen is removed: the leaving animation is finished.
Changed in version 1.6.0: Events on_pre_enter, on_enter, on_pre_leave and on_leave were added.
- manager¶
ScreenManager
object, set when the screen is added to a manager.manager
is anObjectProperty
and defaults to None, read-only.
- name¶
Name of the screen which must be unique within a
ScreenManager
. This is the name used forScreenManager.current
.name
is aStringProperty
and defaults to ‘’.
- transition_progress¶
Value that represents the completion of the current transition, if any is occurring.
If a transition is in progress, whatever the mode, the value will change from 0 to 1. If you want to know if it’s an entering or leaving animation, check the
transition_state
.transition_progress
is aNumericProperty
and defaults to 0.
- transition_state¶
Value that represents the state of the transition:
‘in’ if the transition is going to show your screen
‘out’ if the transition is going to hide your screen
After the transition is complete, the state will retain its last value (in or out).
transition_state
is anOptionProperty
and defaults to ‘out’.
- class kivy.uix.screenmanager.ScreenManager(**kwargs)[source]¶
Bases:
kivy.uix.floatlayout.FloatLayout
Screen manager. This is the main class that will control your
Screen
stack and memory.By default, the manager will show only one screen at a time.
- add_widget(widget, *args, **kwargs)[source]¶
Changed in version 2.1.0: Renamed argument screen to widget.
- clear_widgets(children=None, *args, **kwargs)[source]¶
Changed in version 2.1.0: Renamed argument screens to children.
- current¶
Name of the screen currently shown, or the screen to show.
from kivy.uix.screenmanager import ScreenManager, Screen sm = ScreenManager() sm.add_widget(Screen(name='first')) sm.add_widget(Screen(name='second')) # By default, the first added screen will be shown. If you want to # show another one, just set the 'current' property. sm.current = 'second'
current
is aStringProperty
and defaults to None.
- current_screen¶
Contains the currently displayed screen. You must not change this property manually, use
current
instead.current_screen
is anObjectProperty
and defaults to None, read-only.
- get_screen(name)[source]¶
Return the screen widget associated with the name or raise a
ScreenManagerException
if not found.
- has_screen(name)[source]¶
Return True if a screen with the name has been found.
New in version 1.6.0.
- on_motion(etype, me)[source]¶
Called when a motion event is received.
- Parameters:
- etype: str
Event type, one of “begin”, “update” or “end”
- me:
MotionEvent
Received motion event
- Returns:
bool True to stop event dispatching
New in version 2.1.0.
Warning
This is an experimental method and it remains so while this warning is present.
- on_touch_down(touch)[source]¶
Receive a touch down event.
- Parameters:
- touch:
MotionEvent
class Touch received. The touch is in parent coordinates. See
relativelayout
for a discussion on coordinate systems.
- touch:
- Returns:
bool If True, the dispatching of the touch event will stop. If False, the event will continue to be dispatched to the rest of the widget tree.
- on_touch_move(touch)[source]¶
Receive a touch move event. The touch is in parent coordinates.
See
on_touch_down()
for more information.
- on_touch_up(touch)[source]¶
Receive a touch up event. The touch is in parent coordinates.
See
on_touch_down()
for more information.
- remove_widget(widget, *args, **kwargs)[source]¶
Remove a widget from the children of this widget.
- Parameters:
- widget:
Widget
Widget to remove from our children list.
- widget:
>>> from kivy.uix.button import Button >>> root = Widget() >>> button = Button() >>> root.add_widget(button) >>> root.remove_widget(button)
- screen_names¶
List of the names of all the
Screen
widgets added. The list is read only.screens_names
is anAliasProperty
and is read-only. It is updated if the screen list changes or the name of a screen changes.
- screens¶
List of all the
Screen
widgets added. You should not change this list manually. Use theadd_widget
method instead.screens
is aListProperty
and defaults to [], read-only.
- switch_to(screen, **options)[source]¶
Add a new or existing screen to the ScreenManager and switch to it. The previous screen will be “switched away” from. options are the
transition
options that will be changed before the animation happens.If no previous screens are available, the screen will be used as the main one:
sm = ScreenManager() sm.switch_to(screen1) # later sm.switch_to(screen2, direction='left') # later sm.switch_to(screen3, direction='right', duration=1.)
If any animation is in progress, it will be stopped and replaced by this one: you should avoid this because the animation will just look weird. Use either
switch_to()
orcurrent
but not both.The screen name will be changed if there is any conflict with the current screen.
- transition¶
Transition object to use for animating the transition from the current screen to the next one being shown.
For example, if you want to use a
WipeTransition
between slides:from kivy.uix.screenmanager import ScreenManager, Screen, WipeTransition sm = ScreenManager(transition=WipeTransition()) sm.add_widget(Screen(name='first')) sm.add_widget(Screen(name='second')) # by default, the first added screen will be shown. If you want to # show another one, just set the 'current' property. sm.current = 'second'
transition
is anObjectProperty
and defaults to aSlideTransition
.Changed in version 1.8.0: Default transition has been changed from
SwapTransition
toSlideTransition
.
- exception kivy.uix.screenmanager.ScreenManagerException[source]¶
Bases:
Exception
Exception for the
ScreenManager
.
- class kivy.uix.screenmanager.ShaderTransition[source]¶
Bases:
kivy.uix.screenmanager.TransitionBase
Transition class that uses a Shader for animating the transition between 2 screens. By default, this class doesn’t assign any fragment/vertex shader. If you want to create your own fragment shader for the transition, you need to declare the header yourself and include the “t”, “tex_in” and “tex_out” uniform:
# Create your own transition. This shader implements a "fading" # transition. fs = """$HEADER uniform float t; uniform sampler2D tex_in; uniform sampler2D tex_out; void main(void) { vec4 cin = texture2D(tex_in, tex_coord0); vec4 cout = texture2D(tex_out, tex_coord0); gl_FragColor = mix(cout, cin, t); } """ # And create your transition tr = ShaderTransition(fs=fs) sm = ScreenManager(transition=tr)
- add_screen(screen)[source]¶
(internal) Used to add a screen to the
ScreenManager
.
- clearcolor¶
Sets the color of Fbo ClearColor.
New in version 1.9.0.
clearcolor
is aColorProperty
and defaults to [0, 0, 0, 1].Changed in version 2.0.0: Changed from
ListProperty
toColorProperty
.
- fs¶
Fragment shader to use.
fs
is aStringProperty
and defaults to None.
- remove_screen(screen)[source]¶
(internal) Used to remove a screen from the
ScreenManager
.
- stop()[source]¶
(internal) Stops the transition. This is automatically called by the
ScreenManager
.
- vs¶
Vertex shader to use.
vs
is aStringProperty
and defaults to None.
- class kivy.uix.screenmanager.SlideTransition[source]¶
Bases:
kivy.uix.screenmanager.TransitionBase
Slide Transition, can be used to show a new screen from any direction: left, right, up or down.
- direction¶
Direction of the transition.
direction
is anOptionProperty
and defaults to ‘left’. Can be one of ‘left’, ‘right’, ‘up’ or ‘down’.
- class kivy.uix.screenmanager.SwapTransition(**kwargs)[source]¶
Bases:
kivy.uix.screenmanager.TransitionBase
Swap transition that looks like iOS transition when a new window appears on the screen.
- add_screen(screen)[source]¶
(internal) Used to add a screen to the
ScreenManager
.
- start(manager)[source]¶
(internal) Starts the transition. This is automatically called by the
ScreenManager
.
- class kivy.uix.screenmanager.TransitionBase[source]¶
Bases:
kivy.event.EventDispatcher
TransitionBase is used to animate 2 screens within the
ScreenManager
. This class acts as a base for other implementations like theSlideTransition
andSwapTransition
.- Events:
- on_progress: Transition object, progression float
Fired during the animation of the transition.
- on_complete: Transition object
Fired when the transition is finished.
- add_screen(screen)[source]¶
(internal) Used to add a screen to the
ScreenManager
.
- duration¶
Duration in seconds of the transition.
duration
is aNumericProperty
and defaults to .4 (= 400ms).Changed in version 1.8.0: Default duration has been changed from 700ms to 400ms.
- is_active¶
Indicate whether the transition is currently active or not.
is_active
is aBooleanProperty
and defaults to False, read-only.
- manager¶
ScreenManager
object, set when the screen is added to a manager.manager
is anObjectProperty
and defaults to None, read-only.
- remove_screen(screen)[source]¶
(internal) Used to remove a screen from the
ScreenManager
.
- screen_in¶
Property that contains the screen to show. Automatically set by the
ScreenManager
.screen_in
is anObjectProperty
and defaults to None.
- screen_out¶
Property that contains the screen to hide. Automatically set by the
ScreenManager
.screen_out
is anObjectProperty
and defaults to None.
- start(manager)[source]¶
(internal) Starts the transition. This is automatically called by the
ScreenManager
.
- stop()[source]¶
(internal) Stops the transition. This is automatically called by the
ScreenManager
.
- class kivy.uix.screenmanager.WipeTransition[source]¶
Bases:
kivy.uix.screenmanager.ShaderTransition
Wipe transition, based on a fragment Shader.
- fs¶
Fragment shader to use.
fs
is aStringProperty
and defaults to None.